LangChain in Action: How to Build Intelligent AI Applications Easily and Efficiently ?
Decoding LangChain Parsers: Streamlining AI Data Processing
Welcome to the Ultimate Guide on LangChain Parsers
Dive into the transformative world of LangChain Parsers, where complex data handling meets simplicity and efficiency. This page is your go-to resource for mastering LangChain's powerful parsing capabilities, including String Output Parsers, List Parsers, Regex Parsers, and Custom Output Parsers. Whether you're processing AI-generated text, structuring JSON data, or extracting insights with precision, LangChain Parsers provide the tools you need to streamline workflows and enhance your AI-driven applications. Explore in-depth examples, practical use cases, and easy-to-follow code snippets to unlock the full potential of these advanced parsers. Perfect for developers, data enthusiasts, and AI practitioners alike, this guide is tailored to help you transform, analyze, and simplify data effortlessly.
Let’s simplify AI parsing together!
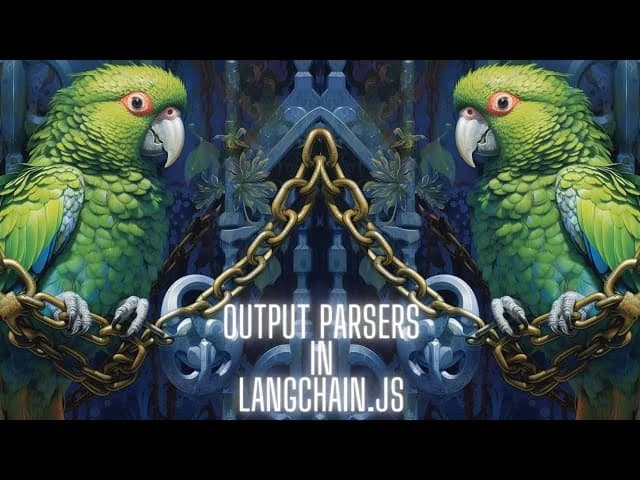
String Output Parser
StringOutputParser is a utility in LangChain that extracts and returns the raw text output from an AI model’s response. It is useful when you want the AI's response as a simple string, rather than as a structured object.
When you call a model using LangChain, the response can be a structured object, sometimes including metadata or formatting. The StringOutputParser simplifies this by extracting only the text content, making it easier to work with.
╔════════════════════════════════════════════════════════════════════════════╗
║ 🌟 EDUCATIONAL EXAMPLE 🌟 ║
║ ║
║ 📌 This is a minimal and short working example for educational purposes. ║
║ ⚠️ Not optimized for production! ║
║ ║
║ 📦 Versions Used: ║
║ - "@langchain/core": "^0.3.38" ║
║ - "@langchain/openai": "^0.4.2" ║
║ ║
║ 🔄 Note: LangChain is transitioning from a monolithic structure to a ║
║ modular package structure. Ensure compatibility with future updates. ║
╚════════════════════════════════════════════════════════════════════════════╝
import { ChatOpenAI } from "@langchain/openai"; // Import the ChatOpenAI class
import { StringOutputParser } from "@langchain/core/output_parsers"; // Import the StringOutputParser class
import dotenv from "dotenv"; // Import the dotenv module
dotenv.config(); // Load environment variables from the .env file
// Initialize the ChatOpenAI model with the required parameters
const model = new ChatOpenAI({
modelName: "gpt-3.5-turbo", // Chat model (gpt-3.5-turbo)
temperature: 0.7, // Adjust the randomness (lower = more factual, higher = more creative)
openAIApiKey: process.env.OPENAI_API_KEY!, // Ensure the API key is loaded
});
// Define a function to run the model
async function run() {
const parser = new StringOutputParser(); // Initialize the StringOutputParser
const prompt = "Provide a brief description of time crystals."; // Define the prompt
const response = await model.invoke(prompt); // Invoke the model with the prompt
let responseText: string; // Initialize a variable to store the response text
if (Array.isArray(response.content)) {
responseText = response.content.map(item => String(item)).join(" ") // Convert array elements to a string
} else {
responseText = String(response.content) // Directly cast to string
}
const parsedOutput = await parser.parse(responseText) // Parse the response content into a clean string
console.log("Parsed Output:", parsedOutput); // Display the parsed output
}
run().catch(console.error); // Call the run function and handle any errors
Where is StringOutputParser Useful?
- Cleaning Up AI Responses Removes extra metadata and extracts just the generated text. Ideal for chatbots, FAQs, or text-based applications.
- Chaining with Other Components Can be combined with prompt templates in a LangChain chain to format AI responses cleanly.
List Output Parser
In AI-driven applications, handling raw text output effectively is crucial, especially when working with structured data. A List Output Parser plays a vital role in converting unstructured AI-generated text into a structured list format that can be easily processed programmatically.
List Output Parsers are particularly useful in scenarios where structured lists are needed, such as:
- Generating recommendations (e.g., “List the top programming languages for AI development”).
- Extracting key points from an AI response (e.g., “Summarize the benefits of TypeScript in bullet points”).
- Creating structured data for further processing in applications (e.g., “List the required skills for a front-end developer”).
- Enhancing AI reliability by enforcing a predictable output format, reducing ambiguity in responses.
By defining a Custom List Output Parser, we ensure that the AI’s response follows a consistent structure, making it more useful for further computation, user interfaces, or integration into automated workflows.
╔════════════════════════════════════════════════════════════════════════════╗
║ 🌟 EDUCATIONAL EXAMPLE 🌟 ║
║ ║
║ 📌 This is a minimal and short working example for educational purposes. ║
║ ⚠️ Not optimized for production! ║
║ ║
║ 📦 Versions Used: ║
║ - "@langchain/core": "^0.3.38" ║
║ - "@langchain/openai": "^0.4.2" ║
║ ║
║ 🔄 Note: LangChain is transitioning from a monolithic structure to a ║
║ modular package structure. Ensure compatibility with future updates. ║
╚════════════════════════════════════════════════════════════════════════════╝
import { ChatOpenAI } from "@langchain/openai"; // import the ChatOpenAI class from the @langchain/openai package
import { ListOutputParser } from "@langchain/core/output_parsers"; // import the ListOutputParser class from the @langchain/core/output_parsers package
import dotenv from "dotenv"; // import the dotenv module
// Load environment variables from the .env file
dotenv.config();
// Initialize the ChatOpenAI model with the required parameters
const model = new ChatOpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY!,
});
// Define a custom ListOutputParser You need to create a CustomListOutputParser because ListOutputParser is an abstract class,
// meaning it cannot be instantiated directly.
class CustomListOutputParser extends ListOutputParser {
lc_namespace = ["custom", "parsers"]; // Namespace
// Override the default parse method
async parse(text: string): Promise<string[]> {
return text.split(",").map((item) => item.trim());
}
// Override the default getFormatInstructions method
getFormatInstructions(): string {
return "Please respond with a comma-separated list of items. Example: Python, JavaScript, Java";
}
}
// Define a function to run the model
async function run() {
const parser = new CustomListOutputParser();
const prompt = `List the top 5 programming languages used in AI development.
${parser.getFormatInstructions()}`;
const response = await model.invoke(prompt); // Invoke the model with the prompt
const responseText = Array.isArray(response.content) ? response.content.join(", ") : response.content; // Convert the response content to a string
console.log("Parsed Output:", responseText);
}
// Call the run function and handle any errors
run().catch(console.error);
It uses OpenAI's GPT-3.5-turbo model to generate responses guided by formatting instructions and processes the output into a structured list. The custom parser is necessary because ListOutputParser is an abstract class, requiring concrete implementation for methods like parse and properties like lc_namespace.
Why we cannot use ListOutputParser directly?
ListOutputParser is abstract and cannot be instantiated. It serves as a blueprint for creating custom parsers by enforcing implementation of required methods and properties. To use it on your webpage, a subclass with these implementations is necessary.
Understanding Structured Output Parsers and Their Use Cases
When working with AI-generated text, ensuring that responses follow a predictable format is essential for automation, data processing, and integration into applications. A structured output parser helps achieve this by transforming unstructured AI responses into well-defined formats like JSON, lists, or key-value pairs.
In this example, the AI is instructed to return information in JSON format, making it easy to parse and use programmatically. Instead of relying on free-text responses, which may vary in structure, a structured output parser ensures that the model consistently produces output that can be processed automatically.
Where Can You Use Structured Output Parsers?
- API Development: Ensure AI-generated responses are formatted as JSON for seamless integration with backend services.
- Data Extraction: Convert unstructured text into structured key-value pairs for easier processing.
- Automation Workflows: Enable AI-driven tools to generate predictable responses that can be used in further operations.
- Chatbots & Virtual Assistants: Maintain structured responses in conversational AI systems to make processing more efficient.
- Report Generation: Standardize outputs for use in documentation, reports, or dashboards.
By leveraging structured output parsers, developers can make AI-generated data more reliable, machine-readable, and easy to integrate into various applications.
╔════════════════════════════════════════════════════════════════════════════╗
║ 🌟 EDUCATIONAL EXAMPLE 🌟 ║
║ ║
║ 📌 This is a minimal and short working example for educational purposes. ║
║ ⚠️ Not optimized for production! ║
║ ║
║ 📦 Versions Used: ║
║ - "@langchain/core": "^0.3.38" ║
║ - "@langchain/openai": "^0.4.2" ║
║ ║
║ 🔄 Note: LangChain is transitioning from a monolithic structure to a ║
║ modular package structure. Ensure compatibility with future updates. ║
╚════════════════════════════════════════════════════════════════════════════╝
import { ChatOpenAI } from "@langchain/openai"; // import the ChatOpenAI class from the @langchain/openai package
import { HumanMessage, SystemMessage } from "@langchain/core/messages"; // import the HumanMessage and SystemMessage classes from the @langchain/core/messages package
import dotenv from "dotenv"; // import the dotenv module
dotenv.config(); // load environment variables from the .env file
// initialize the ChatOpenAI model with the required parameters
const model = new ChatOpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY!,
});
// define a function to run the model
const formatInstructions = `
Provide the following information in JSON format:
{
"name": "string",
"description": "string",
"tags": ["string"]
}
`
// call the run function and handle any errors
async function run() {
// define the messages to send to the model
const msg = [
new SystemMessage("You are a helpful AI that responds with structured JSON."),
new HumanMessage(`Provide information about God's particle:
${formatInstructions}`)
]
const response = await model.invoke(msg) // invoke the model with the messages
const responseText = Array.isArray(response.content) ? response.content.join(" ") : String(response.content) // convert the response content to a string
console.log("Parsed Output:", JSON.parse(responseText)) // log the parsed output
}
// run the function and catch any potential errors
run().catch(console.error);
The parser validates the model's response, enforcing data consistency by checking the types of provided fields such as `name`, `description`, and `tags`. This approach ensures clean and predictable outputs for downstream processing, showcasing the versatility of LangChain's integration for structured data workflows. Ideal for developers seeking robust data parsing in AI-driven applications, this example combines simplicity with precision in handling API responses.
Regex in LangChain
Learn how to extract structured data using regex in LangChain!
╔════════════════════════════════════════════════════════════════════════════╗
║ 🌟 EDUCATIONAL EXAMPLE 🌟 ║
║ ║
║ 📌 This is a minimal and short working example for educational purposes. ║
║ ⚠️ Not optimized for production! ║
║ ║
║ 📦 Versions Used: ║
║ - "@langchain/core": "^0.3.38" ║
║ - "@langchain/openai": "^0.4.2" ║
║ ║
║ 🔄 Note: LangChain is transitioning from a monolithic structure to a ║
║ modular package structure. Ensure compatibility with future updates. ║
╚════════════════════════════════════════════════════════════════════════════╝
import { OpenAI } from "@langchain/openai";
import * as dotenv from "dotenv";
dotenv.config();
async function main() {
const model = new OpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY!,
});
// Define a regex pattern to extract name and age
const regexPattern = /Name:s*([ws]+)s*Age:s*(d+)/;
const prompt = "Provide a fictional character's name and age in the format: 'Name: [name] Age: [age]'.";
const response = await model.call(prompt);
// Apply regex matching manually
const match = response.match(regexPattern);
if (match) {
const parsedOutput = {
name: match[1]?.trim(),
age: match[2]?.trim(),
};
console.log("Parsed Output:", parsedOutput);
} else {
console.error("Failed to parse output with the given regex.");
}
}
main().catch(console.error);
This example demonstrates a manual approach to parsing AI-generated outputs, such as fictional characters' names and ages, into structured objects. Avoiding dependency-specific limitations, this method ensures compatibility and ease of use for a wide range of JavaScript and TypeScript applications. Perfect for parsing formatted AI responses with precision!
Comma-Separated List Output Parser
Discover how to leverage LangChain's CommaSeparatedListOutputParser to structure AI responses into lists for efficient handling.
╔════════════════════════════════════════════════════════════════════════════╗
║ 🌟 EDUCATIONAL EXAMPLE 🌟 ║
║ ║
║ 📌 This is a minimal and short working example for educational purposes. ║
║ ⚠️ Not optimized for production! ║
║ ║
║ 📦 Versions Used: ║
║ - "@langchain/core": "^0.3.38" ║
║ - "@langchain/openai": "^0.4.2" ║
║ ║
║ 🔄 Note: LangChain is transitioning from a monolithic structure to a ║
║ modular package structure. Ensure compatibility with future updates. ║
╚════════════════════════════════════════════════════════════════════════════╝
import { OpenAI } from "@langchain/openai";
import { CommaSeparatedListOutputParser } from "@langchain/core/output_parsers";
import * as dotenv from "dotenv";
dotenv.config();
async function main() {
const model = new OpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY!,
});
const parser = new CommaSeparatedListOutputParser();
const prompt = "List three popular JavaScript frameworks, separated by commas.";
const response = await model.call(prompt);
const parsedOutput = parser.parse(response);
console.log("Parsed Output:", parsedOutput);
}
main().catch(console.error);
This example demonstrates how to integrate OpenAI's GPT-3.5-turbo model to generate a comma-separated list of JavaScript frameworks, and then seamlessly parse the response for immediate use in your applications. Perfect for developers seeking to streamline data extraction in natural language processing tasks.
Custom Output Parser
Unlock the Potential of Custom Output Parsers in LangChain Dive into the world of LangChain with this hands-on example of a Custom JSON Output Parser.
╔════════════════════════════════════════════════════════════════════════════╗
║ 🌟 EDUCATIONAL EXAMPLE 🌟 ║
║ ║
║ 📌 This is a minimal and short working example for educational purposes. ║
║ ⚠️ Not optimized for production! ║
║ ║
║ 📦 Versions Used: ║
║ - "@langchain/core": "^0.3.38" ║
║ - "@langchain/openai": "^0.4.2" ║
║ ║
║ 🔄 Note: LangChain is transitioning from a monolithic structure to a ║
║ modular package structure. Ensure compatibility with future updates. ║
╚════════════════════════════════════════════════════════════════════════════╝
import { OpenAI } from "@langchain/openai";
import { BaseOutputParser } from "@langchain/core/output_parsers";
import * as dotenv from "dotenv";
dotenv.config();
class JSONOutputParser extends BaseOutputParser<any> {
lc_namespace = ["custom", "parsers"];
parse(input: string): any {
try {
return JSON.parse(input);
} catch (error: any) {
throw new Error(`Failed to parse JSON: ${error.message}`);
}
}
getFormatInstructions(): string {
return "Please respond with a valid JSON object. Ensure the syntax is correct.";
}
}
async function main() {
const model = new OpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY!,
});
const parser = new JSONOutputParser();
const prompt = `Describe a programming language in JSON format with fields for "name", "paradigm", and "popularFrameworks".`;
const formattedPrompt = `${prompt}\n\n${parser.getFormatInstructions()}`;
try {
const response = await model.call(formattedPrompt);
console.log("Raw Response:", response);
const parsedOutput = parser.parse(response.trim());
console.log("Parsed Output:", parsedOutput);
} catch (error) {
console.error("Error:", error.message);
}
}
main().catch(console.error);
Learn how to structure your AI responses into usable, structured JSON formats for seamless integration into your applications. This example showcases a practical implementation using LangChain’s powerful output parser base class, helping you transform raw text outputs into precise, structured data. Whether you're building APIs, chatbots, or data-driven apps, this example will elevate your AI parsing capabilities.
Conclusion
LangChain's parsers offer unparalleled flexibility for developers aiming to handle AI-driven data effectively. From simple text transformations to advanced structured JSON responses, these tools streamline data handling processes, ensuring clarity and precision. By incorporating LangChain's parsers into your workflow, you can enhance your AI-powered applications, improving both efficiency and scalability. This page serves as a comprehensive resource for developers to understand and utilize these powerful tools, paving the way for innovative data-driven solutions.