Revolutionizing Document Interaction: Unlocking Insights with LangChain Retrieval Chains
LangChain history refers to the ability of applications powered by LangChain to retain context across conversations. Instead of treating each user query as an isolated event, LangChain's memory components dynamically store and manage past interactions. This enables the creation of intelligent, context-aware systems that deliver seamless and natural conversational experiences, making them ideal for chatbots, virtual assistants, and other interactive applications.
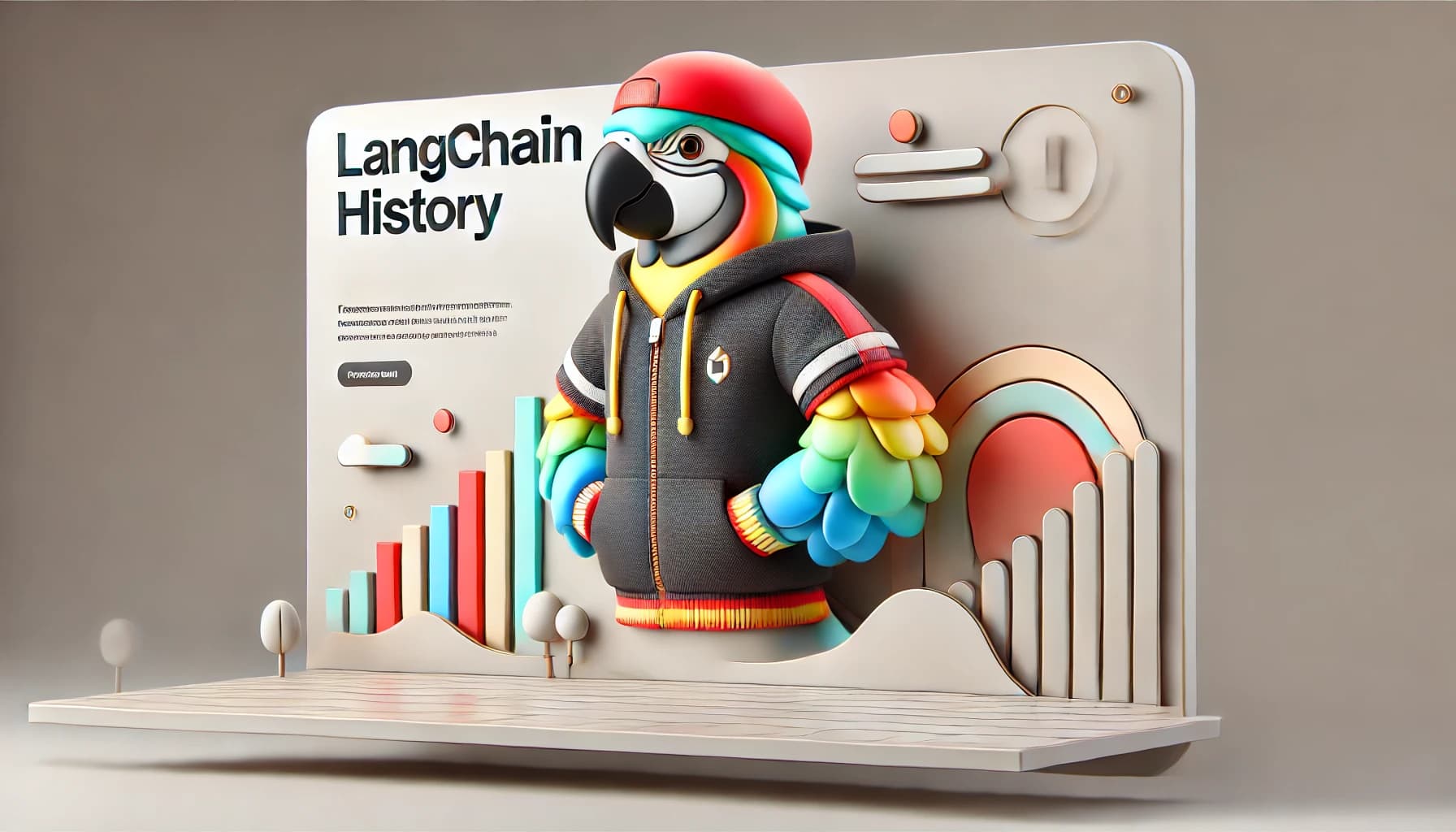
Use Cases
- Chatbots and Virtual Assistants: Retain conversation context to provide coherent, personalized responses. A customer support bot that remembers user preferences or previously reported issues.
- E-Learning Platforms: Track a student’s progress and provide context-aware feedback or follow-up questions. An AI tutor that builds on previous lessons to reinforce learning.
- Dynamic Task Management: Support multi-turn workflows that require remembering intermediate steps. A travel assistant that builds an itinerary over multiple interactions.
- Code Assistants:Retain context about the user’s codebase or project to suggest relevant code snippets. An AI code helper that remembers the programming language and project details.
Enhancing Conversations with LangChain: Chat History in Action
import { ChatOpenAI } from "@langchain/openai";
import { HumanMessage, AIMessage } from "@langchain/core/messages";
import * as dotenv from "dotenv";
dotenv.config();
async function main() {
const chatModel = new ChatOpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY!,
});
const chatHistory = [
new HumanMessage("What is LangChain?"),
new AIMessage("LangChain is a framework for building applications powered by language models."),
new HumanMessage("What are its key features?"),
];
chatHistory.push(new HumanMessage("Can you provide an example of how to use it?"));
const response = await chatModel.call(chatHistory);
console.log("Chat History Example:");
console.log("Response:", response.text || response);
}
main().catch(console.error);
Discover how to build dynamic and context-aware conversations using LangChain's ChatOpenAI module. This method demonstrates creating interactive chat sessions by maintaining a history of user and AI messages. By leveraging LangChain's HumanMessage and AIMessage classes, developers can manage conversational flows, ensuring the model responds contextually to previous interactions. Ideal for building chatbots, virtual assistants, and conversational AI applications, this approach simplifies handling chat histories while ensuring seamless integration with OpenAI's GPT models.
Building Context-Aware Conversations with LangChain's Token Buffer Memory
import { ChatOpenAI } from "@langchain/openai";
import { ConversationChain } from "langchain/chains";
import { ConversationTokenBufferMemory } from "langchain/memory";
import * as dotenv from "dotenv";
dotenv.config();
async function main() {
const chatModel = new ChatOpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY!,
});
// Initialize memory with a token limit
const memory = new ConversationTokenBufferMemory({
llm: chatModel,
maxTokenLimit: 100, // Adjust the token limit as needed
});
const conversation = new ConversationChain({
llm: chatModel,
memory: memory,
});
// Simulate a conversation
console.log(await conversation.call({ input: "What is LangChain?" }));
console.log(await conversation.call({ input: "What are its key features?" }));
console.log(await conversation.call({ input: "Can you provide an example of how to use it?" }));
}
main().catch(console.error);
This code demonstrates the use of LangChain's ConversationTokenBufferMemory to build a context-aware conversational agent. By integrating memory into a conversation chain, the agent retains the history of interactions while managing the memory size by limiting the total token count. The ConversationTokenBufferMemory ensures that only the most relevant parts of the conversation are kept within the specified token limit, optimizing performance and maintaining compatibility with language model constraints. Using OpenAI's GPT-3.5 model (ChatOpenAI), the agent dynamically processes user queries, remembers prior exchanges, and provides context-aware responses. This implementation highlights LangChain's powerful memory management features, enabling the creation of intelligent and seamless conversational experiences for applications like chatbots, virtual assistants, and customer support tools.
Building a Conversational Agent with LangChain’s CombinedMemory
import { ChatOpenAI } from "@langchain/openai";
import { ConversationChain } from "langchain/chains";
import {
CombinedMemory,
ConversationTokenBufferMemory,
ConversationSummaryMemory,
} from "langchain/memory";
import { PromptTemplate } from "@langchain/core/prompts";
import * as dotenv from "dotenv";
dotenv.config();
async function main() {
// Initialize the OpenAI model
const chatModel = new ChatOpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY!,
});
// Initialize individual memory types with unique keys
const tokenBufferMemory = new ConversationTokenBufferMemory({
llm: chatModel,
maxTokenLimit: 150, // Keep recent conversations within 150 tokens
memoryKey: "recent_conversation", // Key for recent conversation memory
});
const summaryMemory = new ConversationSummaryMemory({
llm: chatModel,
memoryKey: "conversation_summary", // Key for summarized memory
});
// Combine the memories with explicit input/output keys
const combinedMemory = new CombinedMemory({
memories: [tokenBufferMemory, summaryMemory],
inputKey: "input", // Explicit input key for ConversationChain
outputKey: "output", // Explicit output key for ConversationChain
});
// Define a custom prompt using PromptTemplate
const prompt = new PromptTemplate({
template: `The following is a conversation:
{conversation_summary}
Recent conversation:
{recent_conversation}
User input:
{input}
AI response:`,
inputVariables: ["conversation_summary", "recent_conversation", "input"], // Variables expected in the prompt
});
// Create a conversation chain with combined memory and custom prompt
const conversation = new ConversationChain({
llm: chatModel,
memory: combinedMemory,
prompt: prompt, // Pass the prompt template
});
// Simulate a conversation
console.log(await conversation.call({ input: "What is LangChain?" }));
console.log(await conversation.call({ input: "What are its key features?" }));
console.log(await conversation.call({ input: "Can you summarize our discussion so far?" }));
}
main().catch(console.error);
This code demonstrates the use of LangChain's CombinedMemory to build a conversational agent that retains both recent and summarized context from a conversation. The example integrates LangChain's ConversationChain with ConversationTokenBufferMemory and ConversationSummaryMemory. The token buffer memory keeps track of recent interactions within a configurable token limit, ensuring that the model has immediate access to the latest details. The summary memory, on the other hand, provides a concise summary of earlier conversations to maintain a broader context without exceeding token limits.
A custom prompt is created using the PromptTemplate class, which incorporates both memory types (recent_conversation and conversation_summary) and the user's current input to guide the model's response. The CombinedMemory is configured to manage these memory components seamlessly. Finally, a ConversationChain ties everything together, using OpenAI's GPT-3.5-turbo model as the language model to handle user inputs and provide contextually rich, dynamic responses.
This implementation highlights how to manage multi-turn conversations effectively while balancing detailed short-term memory with long-term summarized context. It’s ideal for applications like customer support chatbots, educational tutors, and interactive assistants where continuity and memory management are critical.
LangChain Memory Types
Memory Type | Strengths | Limitations |
---|---|---|
Zero-Shot React Description |
|
|
Conversational React Description |
|
|
ConversationTokenBufferMemory |
|
|
ConversationSummaryMemory |
|
|
ConversationBufferMemory |
|
|
CombinedMemory |
|
|
VectorStoreRetrieverMemory |
|
|
ConversationSummaryBufferMemory |
|
|
Conclusion
LangChain offers a robust framework for creating context-aware conversational agents through its versatile memory management capabilities. This tutorial has explored various memory types, including ConversationTokenBufferMemory, ConversationSummaryMemory, and CombinedMemory, demonstrating how they enable seamless integration of short-term and long-term conversation contexts. By leveraging LangChain's tools, such as ChatOpenAI, PromptTemplate, and memory modules, developers can build intelligent applications that adapt dynamically to user interactions while optimizing token usage and performance. These concepts are applicable to a wide range of use cases, from customer support bots and AI tutors to travel planners and code assistants, showcasing LangChain’s potential to revolutionize AI-driven experiences. Whether you're a developer looking to enhance chatbots or create innovative AI solutions, LangChain provides the tools to unlock the full potential of conversational AI.