Revolutionizing Document Interaction: Unlocking Insights with LangChain Retrieval Chains
In the realm of AI-powered applications, retrievals enable seamless access to relevant information from vast collections of unstructured data, such as documents, PDFs, or databases. Instead of treating every query as an isolated event, retrieval mechanisms in LangChain intelligently extract and utilize contextually relevant data to generate more accurate and insightful responses. By leveraging Retrieval Chains, LangChain integrates language models with retrieval systems, creating a powerful synergy for handling complex document interactions.
Use Cases for Retrievals
- Document Search and Summarization: Efficiently find and summarize key information from large document repositories, such as research papers or legal documents.
- Knowledge-Driven Chatbots: Build chatbots capable of answering specific user queries based on company documentation, product manuals, or FAQs.
- Healthcare Record Analysis: Extract insights from patient records to assist medical professionals in diagnosing conditions or reviewing histories.
- E-Learning Platforms: Create personalized study tools by retrieving content from educational materials based on user input.
- Customer Support Automation: Automate support workflows by retrieving relevant troubleshooting steps or policies from internal knowledge bases.
LangChain Retrieval Chains streamline these use cases by allowing applications to retrieve the most relevant information and use it as input for generating meaningful and context-aware responses. This approach not only enhances the efficiency and accuracy of retrieval but also enables the creation of more intelligent and adaptive AI systems.
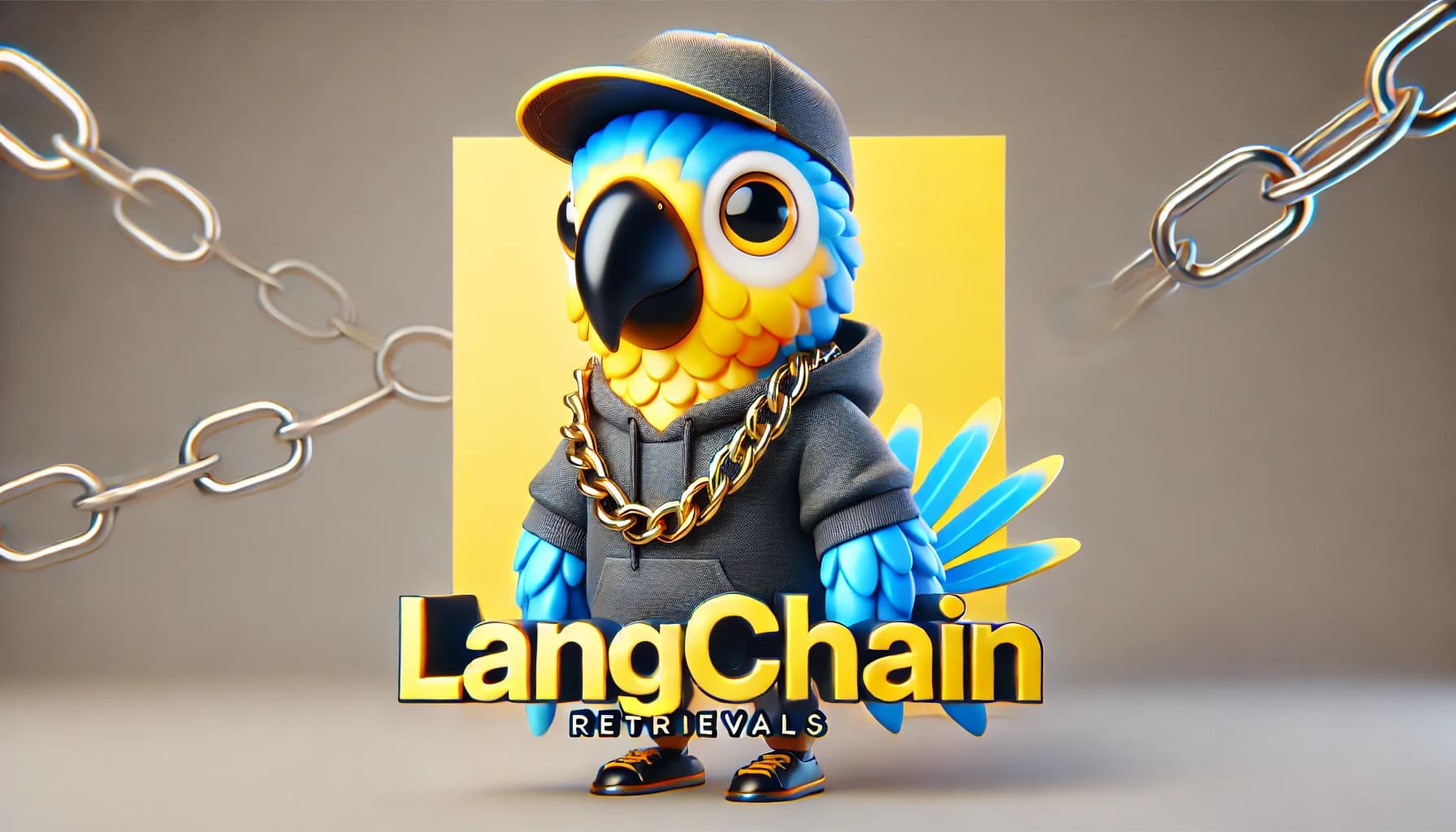
Document Querying
import { ChatOpenAI } from "@langchain/openai";
import { OpenAIEmbeddings } from "@langchain/openai";
import { MemoryVectorStore } from "langchain/vectorstores/memory";
import { RetrievalQAChain } from "langchain/chains";
import * as dotenv from "dotenv";
import * as fs from "fs/promises";
dotenv.config();
async function main() {
try {
const chatModel = new ChatOpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY!,
});
const filePath = "src/sample.txt"; // Ensure the path is correct
const fileContent = await fs.readFile(filePath, "utf8");
console.log("File Content:", fileContent);
const splitDocuments = fileContent.split("
").filter((line) => line.trim() !== "");
console.log("Split Documents:", splitDocuments);
const embeddings = new OpenAIEmbeddings({ openAIApiKey: process.env.OPENAI_API_KEY! });
const vectorStore = await MemoryVectorStore.fromTexts(
splitDocuments, // Use the split lines as documents
splitDocuments.map((_, idx) => ({ id: idx })), // Assign metadata
embeddings
);
console.log("Vector store initialized successfully.");
const retriever = vectorStore.asRetriever({ searchType: "similarity", k: 2 });
const retrievalChain = RetrievalQAChain.fromLLM(chatModel, retriever);
const userQuery = "What is LangChain?";
const response = await retrievalChain.call({ query: userQuery });
const retrievedDocuments = await retriever.getRelevantDocuments(userQuery);
console.log("Retrieved Documents:", retrievedDocuments);
console.log("Response:", response.text);
} catch (error) {
console.error("Error:", error);
}
}
main().catch(console.error);
Explore how to build a document-based Retrieval QA System using LangChain and OpenAI. Learn to leverage MemoryVectorStore and RetrievalQAChain to efficiently process and search text documents. This hands-on tutorial demonstrates how to parse text files, create embeddings, and retrieve answers to user queries using LangChain's powerful framework. Perfect for developers looking to enhance their applications with intelligent document querying and AI-driven insights!
API Retrievals
import { ChatOpenAI } from "@langchain/openai";
import { ChatPromptTemplate } from "@langchain/core/prompts";
import * as dotenv from "dotenv";
import * as readline from "readline";
dotenv.config();
// Initialize readline interface for terminal input
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout,
});
// Function to fetch data from an API using fetch
async function fetchFromAPI(endpoint: string): Promise<any> {
try {
const response = await fetch(endpoint);
// Check if the response is okay
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
// Parse and return the JSON data
return await response.json();
} catch (error) {
// Narrowing the type of the error
if (error instanceof Error) {
console.error("Error fetching data from API:", error.message);
} else {
console.error("Unknown error occurred:", error);
}
return null;
}
}
export async function main() {
// Create the OpenAI model
const model = new ChatOpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY, // Ensure the API key is loaded
});
// Prompt the user for input
rl.question("Enter a user ID to fetch posts: ", async (userId) => {
const apiEndpoint = `https://jsonplaceholder.typicode.com/posts?userId=${userId}`;
console.log("Fetching data from API...");
const apiData = await fetchFromAPI(apiEndpoint);
if (!apiData || apiData.length === 0) {
console.log("No data found for the given user ID.");
rl.close();
return;
}
// Use the retrieved data to generate a response
const prompt = ChatPromptTemplate.fromMessages([
["system", "You are a creative assistant that crafts engaging summaries from API data."],
[
"assistant",
"I will provide a summary of posts retrieved for the given user ID.",
],
[
"human",
"Here are the posts retrieved from the API: {apiData}. Summarize them briefly.",
],
]);
// Format the prompt with the API data
const formattedMessages = await prompt.formatMessages({ apiData: JSON.stringify(apiData, null, 2) });
// Log the conversation flow
formattedMessages.forEach((message) => {
console.log(`[${message._getType().toUpperCase()}]: ${message.content}`);
});
// Generate the summary using the model and the prompt
const response = await model.generate([formattedMessages]);
console.log(`[ASSISTANT]: ${response.generations[0][0].text}`);
// Close the readline interface
rl.close();
});
}
main().catch(console.error);
This code demonstrates how to integrate OpenAI's GPT-3.5 model with the fetch API to retrieve data from a REST API and generate a meaningful summary. It uses the JSONPlaceholder API as a dummy data source to fetch posts based on a user ID. The application guides users through an interactive command-line interface, retrieves posts, and crafts a summary using LangChain's ChatPromptTemplate. It also includes robust error handling for API requests and employs TypeScript for type safety. This example showcases a practical approach to combining API data with AI-powered text generation for building intelligent, interactive applications.
Using MongoDB for Document Retrieval in Node.js
import { MongoClient } from "mongodb";
import * as readline from "readline";
// MongoDB Connection Configuration
const MONGO_URI = "mongodb://localhost:27017";
const DATABASE_NAME = "jobsbg";
const COLLECTION_NAME = "jobs";
// Initialize readline interface for terminal input
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout,
});
async function connectToMongoDB() {
const client = new MongoClient(MONGO_URI);
try {
// Connect to MongoDB
await client.connect();
console.log("Connected to MongoDB at", MONGO_URI);
// Return the database and collection
const database = client.db(DATABASE_NAME);
const collection = database.collection(COLLECTION_NAME);
return { client, collection };
} catch (error) {
console.error("Error connecting to MongoDB:", error);
throw error;
}
}
async function retrieveDocuments(query: object) {
const { client, collection } = await connectToMongoDB();
try {
console.log("Retrieving documents with query:", JSON.stringify(query, null, 2));
// Retrieve documents based on the query
const documents = await collection.find(query).toArray();
console.log("Retrieved Documents:", JSON.stringify(documents, null, 2));
return documents;
} catch (error) {
console.error("Error retrieving documents:", error);
return null;
} finally {
// Close the MongoDB client
await client.close();
console.log("MongoDB connection closed.");
}
}
export async function main() {
rl.question("Enter a key for the query (e.g., title): ", (key) => {
rl.question("Enter a value for the query (e.g., Software Engineer): ", async (value) => {
const query = { [key]: value };
// Retrieve documents based on user input
const documents = await retrieveDocuments(query);
if (!documents || documents.length === 0) {
console.log("No documents found matching the query.");
} else {
console.log("Documents retrieved successfully.");
}
rl.close();
});
});
}
main().catch(console.error);
MongoDB is a powerful NoSQL database, ideal for handling unstructured or semi-structured data. In this example, we demonstrated how to connect to a MongoDB instance using Node.js and retrieve data dynamically from a collection. The script prompts users to input a query key and value, constructs a query object, and fetches matching documents from the specified collection. Leveraging MongoDB’s find method, we retrieve data efficiently and display the results. This approach is perfect for creating interactive tools where users can search large datasets based on flexible criteria. The connection is gracefully handled, ensuring the database remains secure and responsive.
Other Retrieval Chains
- Vector Database Retrieval: Integration with vector stores like Qdrant, Pinecone, Weaviate, Milvus, FAISS, etc., for similarity search. Storing and retrieving embeddings for documents or other data.
- ElasticSearch Retrieval: Use ElasticSearch for retrieving documents based on keyword or full-text search.
- BM25 Retrieval: Classic keyword-based document retrieval using term-frequency inverse document frequency (TF-IDF) or BM25 algorithms.
- SQL Database Retrieval: Query relational databases (e.g., MySQL, PostgreSQL, SQLite) for structured data retrieval.
- Text File or Directory Retrieval: Retrieve documents or data stored in plain text files or a directory.
- Hybrid Search Retrieval: Combines keyword-based and vector-based retrieval for enhanced performance.
- Custom Retrieval: Build your custom retrieval logic by implementing the Retriever interface.
- Google Drive Retrieval: Access documents stored in Google Drive.
- Notion Retrieval: Retrieve data from Notion databases and pages.
- Knowledge Graph Retrieval: Query knowledge graphs like Neo4j.
- Azure Cognitive Search: Azure's full-text search service for enterprise-level data retrieval.
- Document Loaders with Metadata Filtering: Filter and retrieve documents based on metadata tags.
- LangChain Index Retrieval: Use LangChain’s built-in document indexing and retrieval systems.
- ChatGPT Retrieval Plugin: A plugin to connect ChatGPT for retrieving specific datasets.
Conclusion
In the evolving landscape of AI and machine learning, LangChain Retrieval Chains offer an innovative way to bridge the gap between large datasets and intelligent applications. By seamlessly integrating language models with various retrieval mechanisms, LangChain enables developers to unlock valuable insights from structured and unstructured data with unparalleled efficiency.
Through practical examples like document querying and API retrievals, this tutorial highlights how LangChain can transform how we interact with data. These tools simplify complex tasks such as summarizing documents, answering user-specific queries, and retrieving relevant information from diverse sources like vector databases, REST APIs, and more.
The flexibility and scalability of LangChain Retrieval Chains empower developers to create AI-driven applications tailored to unique use cases—whether in healthcare, education, customer support, or beyond. As a result, they pave the way for more interactive, accurate, and adaptive AI systems that revolutionize data interaction and management.
By embracing LangChain, you can harness the power of advanced retrieval chains to build intelligent systems that not only process data but also provide meaningful, actionable insights. The possibilities are endless, and LangChain is your gateway to exploring them.