Unlock Long-Term Memory with LangChain: Persistent Conversations Simplified
In conversational AI, retaining context across interactions is essential for creating personalized and meaningful user experiences. While short-term memory allows AI systems to maintain context within a single session, long-term memory extends this capability, enabling the system to remember information across multiple sessions. LangChain, a powerful framework for building AI applications, offers tools to implement long-term memory by integrating with persistent storage solutions like databases or vector stores. This article explores how LangChain’s long-term memory works, why it’s important, and how it can be used to build smarter, more engaging AI applications.
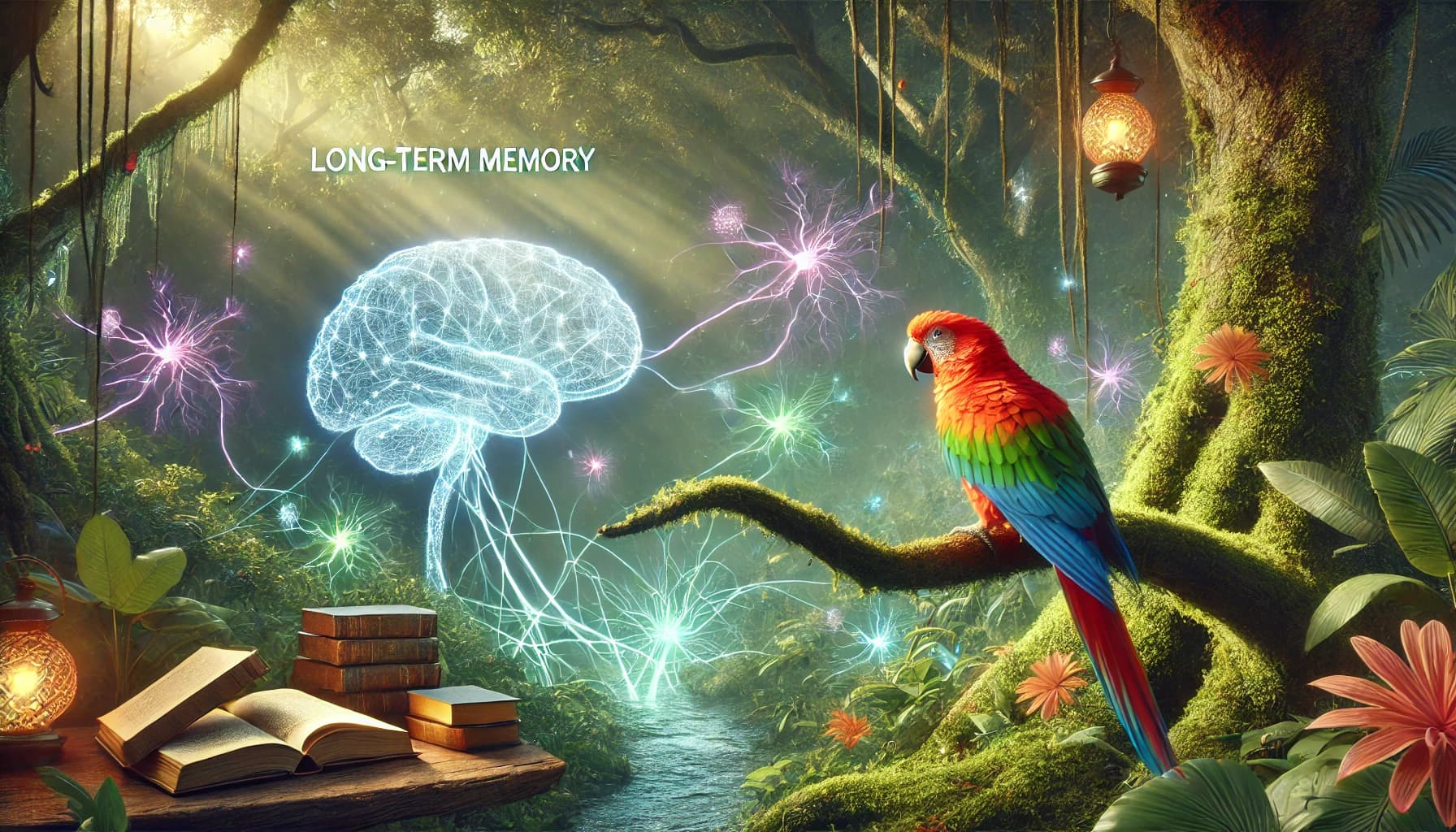
Use Cases
- Personalized Virtual Assistants: Long-term memory allows virtual assistants to remember user preferences, recurring tasks, and past interactions. For instance, a virtual assistant could recall a user's favorite restaurants, preferred travel destinations, or specific scheduling preferences to offer tailored recommendations and reminders.
- Customer Support Systems: AI-driven customer support systems can store and retrieve customer history, such as previous issues, purchase records, or service preferences. This enables agents or AI to provide faster and more relevant assistance by referencing past conversations.
- Educational Tools: Interactive learning platforms can use long-term memory to track a student’s progress, learning preferences, and areas of difficulty. This enables adaptive learning systems to customize lessons, provide targeted exercises, and offer progress reports over time.
- Healthcare Assistants: In medical applications, long-term memory helps store patient histories, such as symptoms, diagnoses, treatments, and test results. Doctors or patients can query the assistant for relevant information, ensuring continuity of care and reducing the need to repeatedly provide the same details.
Database-Persistent Memory
import { ChatOpenAI } from "@langchain/openai";
import { HumanMessage, AIMessage } from "@langchain/core/messages";
import * as dotenv from "dotenv";
import { MongoClient } from "mongodb";
dotenv.config();
const client = new MongoClient(process.env.MONGO_URI!);
const db = client.db("chat_history");
const collection = db.collection("messages");
async function main() {
await client.connect();
const chatModel = new ChatOpenAI({
modelName: "gpt-3.5-turbo",
temperature: 0.7,
openAIApiKey: process.env.OPENAI_API_KEY!,
});
// Fetch previous conversation history from the database
const memoryStore = await collection.find({}).toArray();
// Function to add new messages to the database
async function addToMemory(role: string, content: string) {
await collection.insertOne({ role, content });
}
// New user input
const userInput = "Can you remind me what you said earlier?";
await addToMemory("human", userInput);
// Combine memory store into a format compatible with the model
const conversationHistory = memoryStore.map((msg) =>
msg.role === "human" ? new HumanMessage(msg.content) : new AIMessage(msg.content)
);
// Generate AI response
const response = await chatModel.call(conversationHistory);
// Add the AI response to memory
await addToMemory("ai", response.text || "");
// Output the full conversation
console.log("Conversation History:");
memoryStore.forEach((msg) => {
console.log(`${msg.role.toUpperCase()}: ${msg.content}`);
});
console.log("
AI Response:");
console.log(response.text || response);
await client.close();
}
main().catch(console.error);
This code demonstrates how to create a simple chatbot usingLangChain's ChatOpenAI class with in-memory storage for conversation history. It initializes an AI model (gpt-3.5-turbo) and manages amemoryStore array to keep track of the chat context, storing both human and AI messages. When a user inputs a new message, it is added to the memoryStore, which is then transformed into a format compatible with the model. The AI generates a response based on the conversation history, and this response is appended to the memory for future context. While this setup effectively maintains session-level memory, the conversation history is volatile—it exists only during the program's execution. To extend this into long-term memory, the data would need to be stored in a persistent database or file system. This example highlights the simplicity of managing short-term memory in conversational AI applications.
LangChain Long Term Types
Persistent Memory (Database-Backed Memory)
- Description: This stores conversational history or user data in a persistent storage solution such as a database (e.g., MongoDB, PostgreSQL) or a file system.
- Use Case: Storing structured information like chat logs, user preferences, or task history that can be queried in future sessions.
- Example: A customer support chatbot that remembers previous interactions with users for personalized responses.
Semantic Memory (Vector Store Memory)
- Description: This involves encoding the conversation or data into vector representations using embeddings, then storing these vectors in a vector database (e.g., Pinecone, Qdrant, Weaviate). Retrieval is based on semantic similarity rather than exact matches.
- Use Case: Applications requiring contextual understanding and retrieval of related information, such as knowledge management systems or conversational agents.
- Example: A chatbot that retrieves information about a product based on how semantically similar the user's query is to previously stored information.
Hybrid Memory (Combination of Persistent and Semantic Memory)
- Description: Combines the benefits of persistent (structured) and semantic (unstructured) memory. Conversations or data are stored in both raw (text) and encoded formats for different retrieval needs.
- Use Case: Advanced systems that need to retrieve exact historical context while also retrieving semantically related information.
- Example: An educational platform that can answer direct questions from the course history (persistent) and also suggest related study materials based on semantics.
Episodic Memory
- Description: Focuses on maintaining a snapshot of a specific session or period of interaction. This might not persist indefinitely but is stored long enough for reference within specific contexts.
- Use Case: Applications where continuity within a session or a defined timeframe is important, but long-term retention of data isn’t required.
- Example: A virtual assistant helping with planning an event that remembers details during the planning phase but doesn’t retain them afterward.
Conclusion
LangChain's long-term memory capabilities open up new possibilities for building intelligent and context-aware AI systems. By extending memory beyond a single session, applications can deliver more personalized, efficient, and meaningful interactions. Whether through persistent databases, semantic vector stores, or hybrid approaches, developers have the flexibility to choose a memory type that best suits their use case. As demonstrated, implementing long-term memory not only enhances user experiences but also unlocks the potential for advanced AI-driven applications in areas like customer support, education, healthcare, and beyond. With LangChain, creating persistent and scalable conversational AI solutions has never been more accessible.