React Best Practices - Part 2
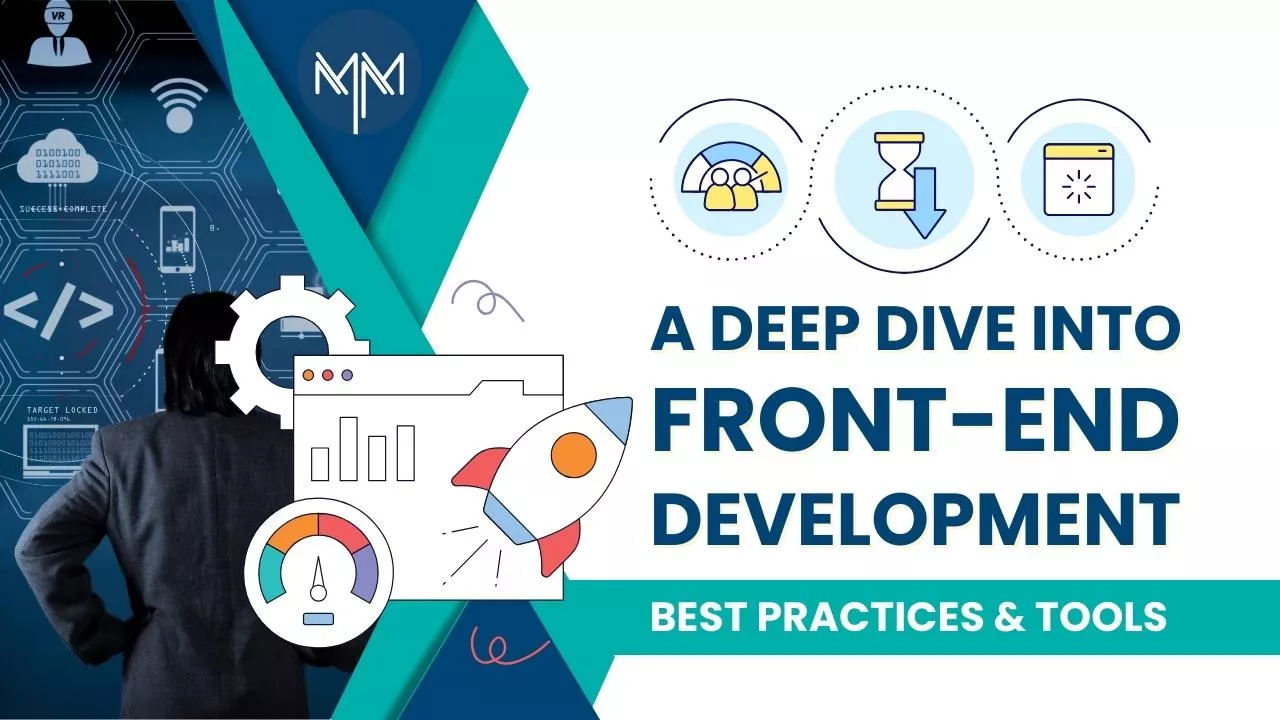
Developing a high-quality frontend application involves more than just writing code that works. It's about building maintainable, scalable, and performant applications that provide a seamless user experience. Adopting best practices ensures that your code remains clean, efficient, and easy to manage as your project grows. From optimizing performance to organizing your codebase effectively, following best practices will help you create frontend applications that are robust, efficient, and easier to maintain in the long run.
Higher-Order Components (HOCs)
Higher-Order Components (HOCs) are a powerful pattern in React used to add or enhance functionality in components. HOCs are functions that take a component as an argument and return a new component with added behavior, without modifying the original component. This pattern promotes code reusability and abstraction, helping developers encapsulate common logic and avoid duplication.
One well-known example of an HOC in React is React.memo() (for React versions less then 19), which enhances a component by memoizing its rendered output, preventing unnecessary re-renders if the props haven't changed.
An HOC is essentially a function that wraps a component and provides additional functionality. This pattern allows you to abstract logic and keep your components clean and focused on their primary role.
For example, React.memo() is a built-in HOC that helps optimize performance by preventing re-renders when a component's props don't change. When React.memo() wraps a component, it only re-renders the component when its props change, thus skipping unnecessary updates.
Without HOC (React.memo)
const Child = ({ name }) => {
console.log('Child component rendered');
return <div>Hello, {name}</div>;
};
const Parent = () => {
const [count, setCount] = React.useState(0);
return (
<div>
<button onClick={() => setCount(count + 1)}>Increment</button>
<Child name="John" />
</div>
);
};
In this example, every time the parent component re-renders (when the button is clicked), the Child component re-renders as well, even though its name prop hasn't changed. This leads to unnecessary rendering.
With HOC (React.memo)
const Child = React.memo(({ name }) => {
console.log('Child component rendered');
return <div>Hello, {name}</div>;
});
const Parent = () => {
const [count, setCount] = React.useState(0);
return (
<div>
<button onClick={() => setCount(count + 1)}>Increment</button>
<Child name="John" />
</div>
);
};
By wrapping Child with React.memo(), React checks whether the name prop has changed before re-rendering the component. In this case, since the name remains the same, the Child component is not re-rendered, leading to better performance.
When to Use HOCs:
- Code Reusability: HOCs allow you to encapsulate shared behavior, such as authentication checks, logging, or performance optimizations, and reuse this logic across multiple components.
- Performance Optimizations: As demonstrated with React.memo(), HOCs can help optimize rendering performance by skipping unnecessary renders.
- Enhanced Functionality: HOCs can add new behavior to components, such as data fetching, handling side effects, or connecting components to external services like Redux.
Benefits of HOCs:
- Abstraction: They help in abstracting complex logic, allowing you to keep components focused on rendering the UI.
- Reuse of Logic: You can reuse common functionality across multiple components without duplicating code.
- Separation of Concerns: HOCs separate the concerns of logic and rendering, making components easier to test and maintain.
In conclusion, Higher-Order Components are a versatile and powerful pattern in React. While they add significant value when needed, it's essential to use them thoughtfully to avoid excessive complexity. React.memo() is a simple and efficient example of how HOCs can be used to optimize React components for better performance.
Error Boundaries
Error boundaries are a crucial feature in React that help catch JavaScript errors anywhere in a component tree, preventing them from crashing the entire app. Instead of the whole UI breaking, error boundaries provide a fallback UI when an error occurs in a component, ensuring a better user experience.
An error boundary is a React component that catches errors during rendering, in lifecycle methods, or in constructors of the child components. When an error is caught, the error boundary can display a fallback UI instead of breaking the whole application. However, error boundaries do not catch errors inside event handlers.
To create an error boundary, you need to use a class component and implement either componentDidCatch() or getDerivedStateFromError() methods.
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render shows the fallback UI
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// You can log the error to an error reporting service
console.log(error, errorInfo);
}
render() {
if (this.state.hasError) {
// You can render any custom fallback UI
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
const ProblematicComponent = () => {
throw new Error('Oops! This component crashed.');
return <div>This will never render.</div>;
};
const App = () => {
return (
<ErrorBoundary>
<ProblematicComponent />
</ErrorBoundary>
);
};
In this example, the ErrorBoundary component catches any error thrown in its child components (like ProblematicComponent). When ProblematicComponent throws an error, the error boundary catches it, preventing the entire app from crashing. Instead, the fallback UI (Something went wrong.) is rendered.
When to Use Error Boundaries:
- Critical Parts of the Application: You should place error boundaries around critical sections of your app, such as components responsible for user input or data fetching, to prevent crashes.
- External API Calls: Use error boundaries to catch errors when components rely on third-party services or APIs, ensuring the app stays functional even if an API fails.
Limitations of Error Boundaries:
- They do not catch errors inside event handlers. You have to handle errors in event handlers manually using try-catch blocks.
- They do not catch errors in asynchronous code (e.g., promises).
Benefits of Error Boundaries:
In summary, error boundaries are an essential feature for building resilient React applications. By implementing error boundaries, you can catch and handle errors more gracefully, ensuring that users have a smoother and more consistent experience, even when something goes wrong.
Suspense
Suspense is a powerful feature in React that allows you to manage asynchronous operations, like code-splitting and data fetching, while providing a fallback UI during the waiting period. It is primarily used in conjunction with React.lazy() for lazy loading components and is also a core part of React's concurrent rendering model.
Suspense lets you show a fallback UI (like a loading spinner or message) while waiting for a component to load or some asynchronous operation to complete. When the asynchronous operation finishes, the Suspense component renders the actual content.
Currently, Suspense is widely used for lazy-loading components with React.lazy(), but React is expanding its use cases to include data fetching with libraries like React Query or Relay.
Lazy Loading with Suspense:
import React, { Suspense, lazy } from 'react';
// Lazy load a component
const LazyComponent = lazy(() => import('./LazyComponent'));
const App = () => {
return (
<div>
<h1>My App</h1>
<Suspense fallback={<div>Loading component...</div>}>
<LazyComponent />
</Suspense>
</div>
);
};
export default App;
In this example:
- LazyComponent is loaded only when it is needed, using React.lazy().
- Suspense provides a fallback UI (Loading component...) while LazyComponent is being fetched.
- Once the component is loaded, LazyComponent is rendered in place of the fallback.
When to Use Suspense:
- Lazy Loading Components: When you don’t want to load large components or entire pages until they’re needed, use Suspense with React.lazy(). This improves performance by reducing the size of the initial JavaScript bundle.
- Handling Asynchronous Data: Although not fully implemented yet, future React features will allow Suspense to handle asynchronous data fetching, letting components render the UI only after the required data is available.
- Rendering Delays: Use Suspense to manage components that may take some time to render, providing users with feedback (such as a loading indicator) during the delay.
Benefits of Suspense:
- Improved User Experience: By providing a fallback UI during loading states, Suspense improves user experience, preventing the app from appearing unresponsive or blank while waiting for asynchronous tasks.
- Simpler Asynchronous Logic: Suspense simplifies code handling for asynchronous operations like lazy loading, hiding complex logic behind an easy-to-use component interface.
- Concurrent Rendering Ready:Suspense is a crucial part of React’s future concurrent rendering features, ensuring your app can remain responsive even with large-scale updates.
Future Use Cases:
With future versions of React, Suspense will also support asynchronous data fetching. This will allow you to wrap components that depend on data fetching in a Suspense boundary, showing fallback UIs until the data is fully loaded.
For example, with the upcoming integration of Suspense with data-fetching libraries:
<Suspense fallback={<div>Loading data...</div>}>
<UserProfile />
</Suspense>
In this case, Suspense could be used to wrap UserProfile, showing a loading spinner until all the required data is fetched and the component is ready to render.
In conclusion, Suspense is a versatile tool that enhances user experience by allowing you to handle asynchronous component loading more gracefully. As React continues to evolve, its role will expand to cover even more advanced use cases like data fetching and concurrent rendering.
Abort Controller
AbortController is a browser-native API used to abort asynchronous requests, such as fetch requests, in JavaScript. In React, it’s particularly useful when dealing with long-running or redundant API requests. If a component unmounts before a request completes or if a new request is triggered before the previous one finishes, you can use AbortController to cancel the in-flight request, preventing memory leaks or unnecessary operations.
When working with asynchronous requests in React, it's common to fetch data when a component mounts. However, there are cases where the component may unmount before the request finishes (e.g., when navigating to another page). If the request completes after the component has unmounted, trying to update the state of the unmounted component could cause errors and memory leaks.
By using AbortController, you can cancel the ongoing fetch request if the component unmounts, ensuring the app doesn’t try to update the state of an unmounted component.
Using AbortController in a React Component:
import React, { useState, useEffect } from 'react';
const DataFetcher = () => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const controller = new AbortController(); // Create an AbortController instance
const signal = controller.signal;
const fetchData = async () => {
try {
const response = await fetch('https://api.example.com/data', { signal });
const result = await response.json();
setData(result);
} catch (err) {
if (err.name !== 'AbortError') {
setError('Failed to fetch data');
}
} finally {
setLoading(false);
}
};
fetchData();
// Clean up function: abort the fetch request if the component unmounts
return () => {
controller.abort();
};
}, []); // Empty dependency array means the effect runs on mount
if (loading) return <div>Loading...</div>;
if (error) return <div>{error}</div>;
return <div>{JSON.stringify(data)}</div>;
};
export default DataFetcher;
- AbortController is created at the start of the useEffect hook.
- The fetch request includes an AbortController signal (signal), which allows the fetch to be aborted if needed.
- If the component unmounts before the request completes, the clean-up function is triggered, and the fetch is aborted using controller.abort().
- If the fetch is aborted, the catch block checks if the error is an AbortError to prevent displaying unnecessary error messages.
Benefits of Using AbortController:
- Prevents Memory Leaks: By aborting requests when a component unmounts, you avoid trying to update the state of unmounted components, preventing memory leaks and errors.
- Efficient Resource Management: It helps manage network resources by stopping redundant or outdated requests when newer requests are triggered.
- Better User Experience: Users can navigate away from or re-trigger actions (e.g., re-fetching data) without performance degradation or waiting for previous operations to complete.
Use Cases for AbortController:
- Component Unmounting: When a user navigates away from a component that triggers a fetch request, you should abort the ongoing request to prevent unneeded state updates.
- Redundant API Calls: If a user triggers multiple requests (e.g., a search feature), you can cancel previous requests to avoid redundant fetches and improve performance.
In summary, AbortController is a valuable tool when working with asynchronous requests in React. It ensures efficient resource management by allowing you to abort requests when components unmount or when newer requests take priority.
Separation of concerns
Separation of concerns is a fundamental design principle that helps keep your code organized, modular, and maintainable. In React, this means splitting your code into distinct sections or components, each responsible for a specific part of your application’s functionality. By ensuring each component or function only handles one responsibility, you reduce complexity, enhance reusability, and make your codebase easier to maintain and scale.
In React, separating concerns often means:
- Breaking down large components into smaller, more manageable ones.
- Isolating business logic from the UI by keeping complex logic out of your presentational components.
- Grouping related concerns such as data fetching, state management, and event handling into appropriate components or hooks.
By separating concerns, each component or function becomes more focused and easier to understand, test, and debug. It also promotes reusability, as smaller components with clear responsibilities can be easily reused across the app.
Without Separation of Concerns (Monolithic Component):
import React, { useState, useEffect } from 'react';
const UserProfile = () => {
const [user, setUser] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
const fetchUser = async () => {
const response = await fetch('/api/user');
const data = await response.json();
setUser(data);
setLoading(false);
};
fetchUser();
}, []);
if (loading) return <div>Loading...</div>;
return (
<div>
<h1>{user.name}</h1>
<p>{user.email}</p>
</div>
);
};
In this example, the UserProfile component is responsible for fetching data, managing state, and rendering the UI. This makes the component harder to maintain and less reusable.
With Separation of Concerns (Modular Approach):
import { useState, useEffect } from 'react';
export const useFetchUser = () => {
const [user, setUser] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
const fetchUser = async () => {
const response = await fetch('/api/user');
const data = await response.json();
setUser(data);
setLoading(false);
};
fetchUser();
}, []);
return { user, loading };
};
User Profile UI Component:
import React from 'react';
import { useFetchUser } from './useFetchUser';
const UserProfile = () => {
const { user, loading } = useFetchUser();
if (loading) return <div>Loading...</div>;
return (
<div>
<h1>{user.name}</h1>
<p>{user.email}</p>
</div>
);
};
export default UserProfile;
- The useFetchUser custom hook isolates the data fetching logic.
- The UserProfile component is solely responsible for rendering the UI based on the fetched data.
This makes the code more modular and easier to maintain, as the data fetching logic can now be reused by other components that need user data, and the UI component is simpler and easier to reason about.
Benefits of Separating Concerns:
- Modularity and Reusability: Smaller, focused components and hooks can be reused in different parts of the app, reducing duplication and increasing consistency.
- Easier Maintenance: Components that follow separation of concerns are easier to maintain because each part has a clear responsibility. This makes it simpler to update, debug, and test the app.
- Improved Testability: By isolating logic from the UI, you can write more focused and effective unit tests, improving the overall quality and robustness of the application.
- Scalability: As your app grows, separating concerns makes it easier to scale the codebase. Different teams or developers can work on distinct parts of the application without stepping on each other’s toes.
In conclusion, separating concerns in React leads to a more maintainable, modular, and testable codebase. It encourages clean, reusable components and simplifies complex applications by distributing responsibilities into focused, manageable parts.
Conclusion
In developing high-quality frontend applications, adhering to best practices is essential for building scalable, maintainable, and performant solutions. Techniques like utilizing Higher-Order Components (HOCs), implementing error boundaries, leveraging Suspense for asynchronous operations, and managing resources efficiently with AbortController are vital to optimizing React applications. Furthermore, adhering to the principle of separating concerns keeps your code modular, easier to maintain, and more reusable, contributing to a cleaner, more organized codebase. By adopting these best practices, developers can create robust and efficient frontend applications that deliver a superior user experience while ensuring long-term maintainability and scalability.