Essential Security Practices for a Secure Node.js Application
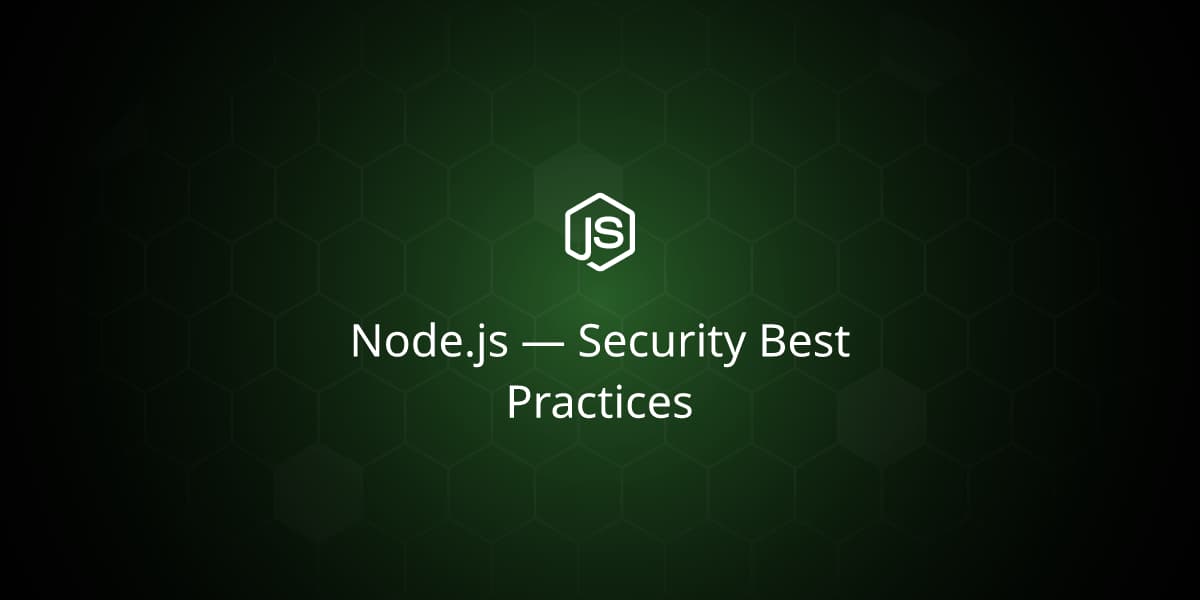
Ensuring the security of a Node.js application involves implementing various security measures to protect against common vulnerabilities and attacks. This article highlights crucial security practices, tools, and configurations to secure your application effectively.
Visit 'JobApi Application' with Express and TypeScript and download the code from GitHub to see how these security practices are implemented
Helmet for Security Headers
Helmet is a middleware for Express applications that helps secure your app by setting various HTTP headers. It mitigates common security vulnerabilities such as cross-site scripting (XSS) and clickjacking.
Key Features:- XSS Protection: Prevents cross-site scripting attacks.
- Clickjacking: Secures the app against clickjacking attacks.
- Other Headers: Sets other important security headers like X-Content-Type-Options, Strict-Transport-Security, and more.
- Content Security Policy (CSP)
CSURF - Cross-Site Request Forgery
Cross-Site Request Forgery (CSRF) is a type of attack that tricks the victim into submitting a malicious request. It exploits the trust that a site has in the user's browser. To protect your application from CSRF attacks, you can use the csurf middleware in your Express applications.
The csurf middleware generates a unique CSRF token that is required to be sent with any state-changing request (like POST, PUT, DELETE). This token ensures that the request comes from a trusted source.
CORS - Cross-Origin Resource Sharing
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to control how resources on a web server are requested from another domain, protocol, or port than the server itself. In a Node.js application, configuring CORS properly is crucial to ensure that your resources are accessed securely and only by trusted origins.
CORS works by adding HTTP headers that tell browsers to allow web applications running at one origin to access resources from a different origin. This helps to prevent certain types of attacks, such as Cross-Site Request Forgery (CSRF) and Cross-Site Scripting (XSS), by ensuring that only specified origins are allowed to access your server's resources.
Sanitizing Requests
Sanitizing requests is a critical step in protecting your application from cross-site scripting (XSS) and SQL injection attacks. One effective way to sanitize user inputs in a Node.js application is by using the DOMPurify library. DOMPurify is a DOM-only, super-fast, uber-tolerant XSS sanitizer for HTML, MathML, and SVG.
- Robust Protection: DOMPurify provides strong protection against XSS attacks by sanitizing potentially malicious HTML and scripts.
- Speed: It is optimized for performance, making it suitable for real-time sanitization.
- Compatibility: Works well with different environments, including Node.js.
Implementing rate limiting to protect from excessive requests
Rate limiting is a crucial security measure to protect your Node.js application from excessive requests, which can lead to denial-of-service (DoS) attacks or abuse. By limiting the number of requests, payload size, and implement express-slow-down (If a client sends more than 100 requests within a 15-minute window, their subsequent requests will be delayed by 500ms for each additional request.) a client can make within a certain timeframe, you can ensure that your server remains available and responsive for legitimate users.
Customizing Rate Limiting- windowMs: The time frame for which requests are checked/remembered.
- max: The maximum number of requests allowed within the windowMs time frame.
- message: The response message sent when the rate limit is exceeded.
- headers: Whether to add X-RateLimit-* headers to the response (true by default).
- Dynamic Limits: Set dynamic limits based on user roles or other criteria.
- Prevents Abuse: Protects your server from being overwhelmed by too many requests.
- Improves Stability: Ensures that resources are available for legitimate users.
- Enhances Security: Mitigates the risk of DoS attacks.
HTTPS Enforcement
Enforcing HTTPS in your Node.js application is crucial to ensure the security of data in transit. HTTPS encrypts the data exchanged between the client and the server, protecting it from interception and tampering.
Why Enforce HTTPS?- Data Encryption: Protects sensitive data such as login credentials, personal information, and payment details.
- Integrity: Ensures that the data sent and received has not been altered.
- Authentication: Confirms the identity of the website, preventing man-in-the-middle attacks.
- SEO Benefits: Search engines favor HTTPS-enabled sites, improving your search rankings.
Dependency Security
Third-party dependencies can contain vulnerabilities that, if exploited, can compromise your application. Ensuring that all dependencies are secure and up-to-date helps mitigate these risks.
Best Practices for Dependency Security- Regularly Update Dependencies: Keeping your dependencies up-to-date ensures you benefit from the latest security patches and bug fixes.
- Use Trusted Sources: Only use libraries from trusted and well-maintained sources to minimize the risk of incorporating vulnerable code.
- Monitor for Vulnerabilities: Continuously monitor your dependencies for known vulnerabilities and address them promptly.
- npm audit is a built-in tool for Node.js that analyzes your project's dependencies for security vulnerabilities.
- Snyk is a powerful tool that finds and fixes vulnerabilities in your dependencies. It offers continuous monitoring and can be integrated into your development workflow.
- Dependabot - Dependabot automatically checks for dependency updates and creates pull requeststo update them.
- Renovate is a tool that automates dependency updates and provides comprehensive configuration options.
JWT Security: Best Practices for Securing JSON Web Tokens
JSON Web Tokens (JWT) are a popular method for securely transmitting information between parties as a JSON object. They are widely used for authentication and information exchange. However, to ensure the security of your application, it is essential to implement best practices when using JWTs.
A JWT consists of three parts:- Header: Contains the type of token (JWT) and the signing algorithm.
- Payload: Contains the claims, which are statements about an entity (typically, the user) and additional data.
- Signature: Used to verify the token's integrity and authenticity.
- Use a Strong Secret Key - The secret key used to sign the JWT should be complex and kept confidential. A strong key ensures that the token cannot be easily tampered with.
- Set Appropriate Expiration Times - Always set an expiration time (exp) for your JWTs to limit the window of opportunity for an attacker to misuse the token if it is compromised.
- Use HTTPS to Secure Data in Transit - Ensure that your application enforces HTTPS to protect tokens from being intercepted during transmission.
- Validate JWTs on the Server - Always validate the JWT on the server to ensure its integrity and authenticity. Use a library like jsonwebtoken to verify tokens.
- Use Appropriate Algorithms - Use strong and recommended algorithms for signing the JWT. Avoid using none or weak algorithms, Recommended Algorithms: HS256, RS256.
- Implement Token Rotation - Token rotation involves issuing new tokens frequently and invalidating old ones. This reduces the risk associated with long-lived tokens.
- Store Tokens Securely - Store tokens securely on the client side. For web applications, use HTTP-only and secure cookies to store JWTs.
- Regularly Rotate Signing Keys - Regularly rotate your signing keys to reduce the impact of a compromised key. Implement a key rotation strategy and ensure backward compatibility during the transition period.
- Monitor and Revoke Compromised Tokens - Implement mechanisms to monitor and revoke tokens that are suspected of being compromised. Maintain a blacklist of revoked tokens and check against it during token validation.
- Implement Audience and Issuer Claims - Use the aud (audience) and iss (issuer) claims to ensure that the token is intended for your application and issued by a trusted source.
Database Security: Essential Practices for Securing Your Database
Securing your database is a critical aspect of ensuring the overall security of your application. A well-secured database prevents unauthorized access, data breaches, and protects sensitive information from various types of attacks.
Here are essential practices for database security:- Use Prepared Statements and ORM Libraries - Using prepared statements and Object-Relational Mapping (ORM) libraries helps prevent SQL injection attacks. Prepared statements ensure that SQL queries are properly parameterized, while ORM libraries provide an abstraction layer that handles query building and execution securely.
- Escape Queries - When executing raw SQL queries, ensure that all inputs are properly escaped to prevent injection attacks. Many database libraries provide methods for escaping inputs.
- Enable Encryption-at-Rest - Encrypting your database at rest ensures that data is protected even if the physical storage media is compromised. Most modern database management systems offer built-in support for encryption-at-rest.
- Implement Access Controls - Restrict access to the database by implementing role-based access controls (RBAC). Ensure that only authorized users and services can access the database and perform specific actions.
- Regularly Update and Patch - Keep your database software up to date with the latest patches and updates. Regular updates address security vulnerabilities and improve the overall security of the system.
- Backup Data Securely - Regularly back up your database and ensure that backups are stored securely. Encrypt backups to protect data and verify the integrity of backup files.
- Use Strong Authentication Mechanisms - Implement strong authentication mechanisms to secure access to the database. Use multi-factor authentication (MFA) where possible and enforce strong password policies.
- Monitor and Audit Database Activity - Set up logging and monitoring to track database activity. Regularly review logs for suspicious activities and configure alerts for potential security incidents.
- Secure Configuration - Ensure that your database is securely configured. Disable default accounts, change default passwords, and remove unnecessary services or features.
- Use Secure Connections - Always use secure connections (TLS/SSL) to encrypt data transmitted between your application and the database. This protects data from being intercepted during transit.
- Implement Least Privilege - Apply the principle of least privilege by granting users the minimum level of access required to perform their tasks. Avoid using administrative accounts for routine operations.
- Regular Security Audits - Conduct regular security audits and vulnerability assessments to identify and remediate potential security issues in your database configuration and usage.
Logging and Monitoring for Secure Node.js Applications
Logging and monitoring are critical components for maintaining the security and performance of your Node.js application. Effective logging helps you track user activities and system events, while monitoring allows you to detect and respond to security incidents and performance issues in real-time.
Why Logging and Monitoring Are Important- Security: Detect and respond to suspicious activities, potential breaches, and vulnerabilities.
- Performance: Monitor application performance and identify bottlenecks or issues.
- Compliance: Maintain logs for compliance with legal and regulatory requirements.
- Debugging: Facilitate troubleshooting and debugging by providing detailed records of application behavior.
Integrate your application with a monitoring service such as New Relic, Datadog, or Prometheus. These services provide real-time monitoring, alerting, and dashboards.
Effective Session Management in Node.js Applications
Session management is a critical aspect of securing Node.js applications, particularly those that involve user authentication and maintaining state across multiple requests. Proper session management ensures that user sessions are handled securely, reducing the risk of session hijacking and other related attacks. Here’s a detailed guide on implementing secure session management in your Node.js application.
- Use Secure Cookies - Cookies are commonly used to store session IDs. Ensuring these cookies are secure is paramount.
- Proper Session Expiration - Set appropriate expiration times for sessions to minimize the risk of long-lived sessions being hijacked.
- Implement Session Regeneration - Regenerate session IDs after a successful login to prevent session fixation attacks.
- Use Strong Session Secrets - Use a strong and unpredictable secret key for signing the session ID cookie. Avoid using hardcoded or weak secrets.
- Store Sessions Securely - Consider storing session data in a secure store such as a database or an in-memory store like Redis, especially for distributed applications.
- Implement Logout Functionality - Ensure users can log out effectively, destroying their session and cookies.
- Monitor and Limit Session Usage - Track session activity and limit the number of active sessions per user to prevent abuse.
- Secure Session Data - Encrypt session data if it contains sensitive information and ensure that data is properly validated and sanitized.
Error Handling
Proper error handling is crucial for maintaining application security. Poor error handling can expose sensitive information about your system, making it easier for attackers to exploit vulnerabilities. Here are the best practices for error handling in a secure Node.js application:
- Generic Error Messages - Ensure that error messages sent to clients do not leak sensitive information about the server's architecture, stack traces, or database schema.
- Logging Detailed Errors - While clients should receive generic messages, detailed error information should be logged internally. This helps in diagnosing issues without exposing critical information.
- Client-Side Error Handling - Implement error handling on the client side to gracefully manage errors and provide a better user experience.
- Validation Errors - For validation errors, provide specific but non-revealing messages to help users correct their input without exposing internal logic.
- Security-Specific Errors - Handle security-specific errors, such as authentication and authorization failures, without revealing details about the authentication mechanism.