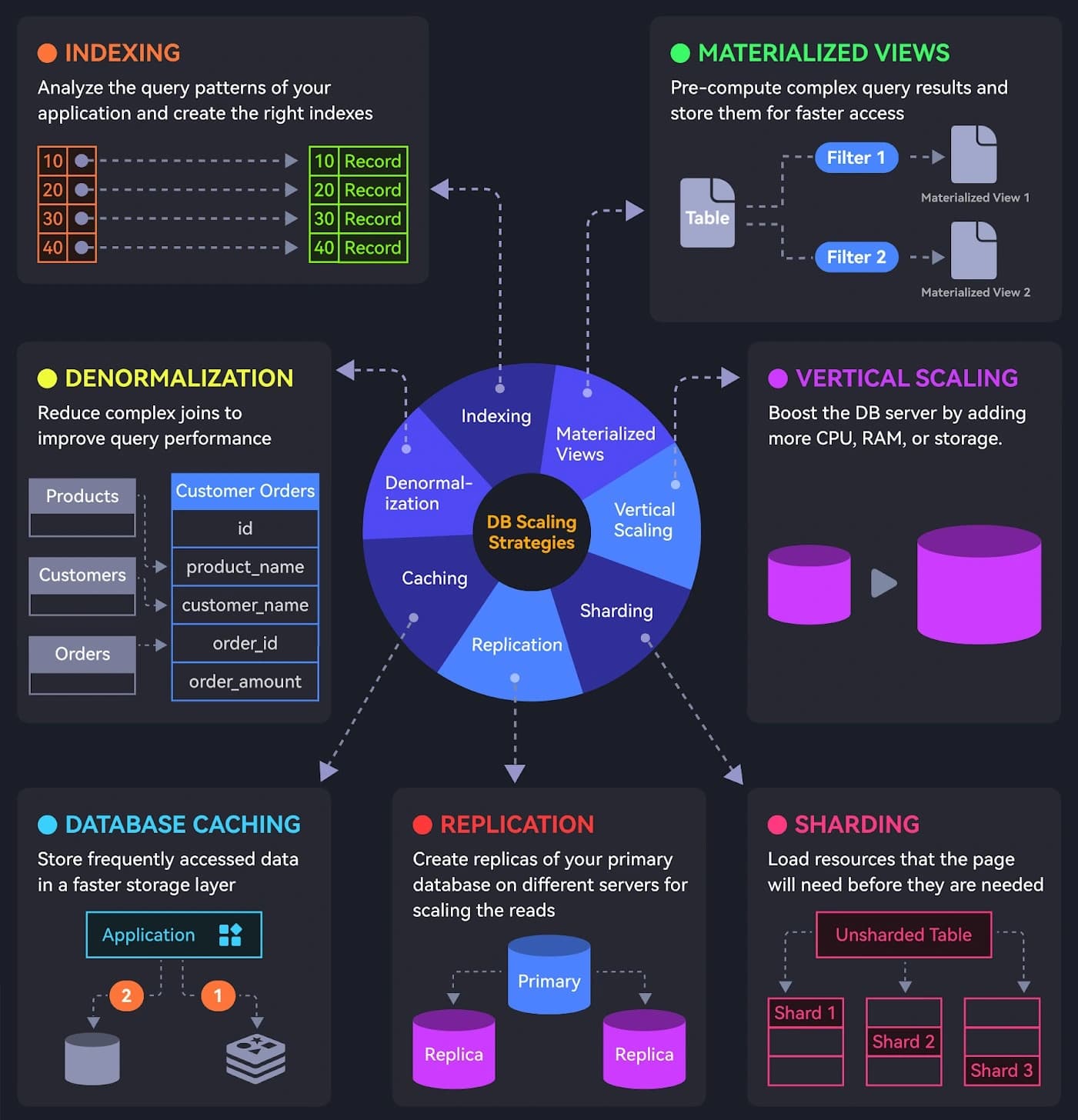
Scaling a database effectively is crucial for handling increased data volume, user load, and maintaining performance and availability. Here are strategies that can help scale a database depending on specific requirements and growth stages:
Relative Links
Optimize Database Queries
Optimize Database Queries is a crucial concept in database management that focuses on improving the efficiency and speed of data retrieval processes, which can greatly impact the performance of applications that rely on database interactions. Here’s a breakdown of what it involves:
- Efficient Query Design: Writing well-structured queries with minimizing data transfer helps in reducing the load on the database. Queries should be as specific as possible to avoid retrieving unnecessary data, which saves time and resources.
Bad ExampleSELECT * FROM users WHERE age = 25;
Good ExampleSELECT name, email FROM users WHERE age = 25;
- Avoiding Complex Joins and Nested Queries: Joins and nested queries are sometimes necessary, but they can be resource-intensive. Simplifying queries or breaking them into smaller, more manageable parts can help reduce complexity.
Scenario: Suppose we have two tables in an e-commerce database:- Customers: Stores customer information.
- Orders: Stores orders with a reference to customer_id.
Bad ExampleSELECT c.customer_id, c.customer_name FROM Customers c JOIN (SELECT customer_id FROM Orders WHERE order_date >= NOW() - INTERVAL '30 days') o ON c.customer_id = o.customer_id;
Good ExampleSELECT DISTINCT c.customer_id, c.customer_name FROM Customers c JOIN Orders o ON c.customer_id = o.customer_id WHERE o.order_date >= NOW() - INTERVAL '30 days';
- Using Appropriate Filtering and Sorting: Filtering results through WHERE clauses or sorting with ORDER BY can help narrow down the data the database needs to process. However, overuse of filters and sorts without indexes can slow down performance.
Scenario: Retrieve all employees in the “Sales” department, sorted by salary (highest to lowest).
Bad ExampleSELECT * FROM employees ORDER BY salary DESC;
Good Example
By applying filtering (WHERE department = 'Sales') first, the query becomes more efficient, focusing on a smaller subset of relevant data before sorting, which improves overall speed.SELECT * FROM employees WHERE department = 'Sales' ORDER BY salary DESC;
- Limiting Result Sets: Using limits (e.g.,LIMIT in SQL) is especially helpful when only a small subset of the data is needed, reducing memory and CPU usage.
Scenario: Imagine you have a users table with thousands of records, and you want to fetch the top 10 newest users.
Bad ExampleSELECT * FROM users ORDER BY created_at DESC;
Good Example
Using LIMIT in the query helps control the number of rows returned, improving query performance, especially in tables with large datasets.SELECT * FROM users ORDER BY created_at DESC LIMIT 10;
Indexing
What Indexes Do: Indexes are data structures that help the database find and retrieve specific records faster by providing shortcuts to the data, much like an index in a book.
Types of Indexes: Databases can have different types of indexes, such as:
single-field indexes,
CREATE INDEX idx_last_name ON users(last_name);
====
//Speeds up queries like:
====
SELECT * FROM users WHERE last_name = 'Smith';
composite indexes (across multiple columns)
CREATE INDEX idx_customer_order_date ON orders(customer_id, order_date);
====
//Speeds up queries like:
====
SELECT * FROM orders WHERE customer_id = 123 AND order_date = '2024-01-01';
and full-text indexes
CREATE FULLTEXT INDEX idx_content ON posts(content);
====
//Speeds up queries like:
====
SELECT * FROM posts WHERE MATCH(content) AGAINST('database indexing');
Each has its use case depending on the type of queries.
- Indexing Frequently Queried Fields: By creating indexes on fields that are often used in WHERE, JOIN, and ORDER BY clauses, you can dramatically speed up queries on those fields.
- Balancing Indexes and Write Performance: While indexes improve read operations, they can slow down write operations (e.g.,INSERT, UPDATE, DELETE) because the index also needs to be updated. Balancing indexing for read-heavy vs. write-heavy applications is important.
Example: Indexing for Read-Heavy Application Reporting system where users frequently query sales data by sale_date.
CREATE INDEX idx_sale_date ON sales(sale_date);
- Read Performance: Greatly improves read performance by allowing fast lookups on sale_date for reporting.
- Write Performance: Every time a new sale is inserted, the index on sale_date must also be updated, slightly slowing down INSERT operations.
- Best for: Read-heavy applications, where fast querying outweighs the impact of slower inserts.
Example for Write-Heavy Application Real-time data collection system where new sales are recorded frequently.Avoiding Over-Indexing: Too many indexes can bloat storage and degrade write performance. It’s best to index only the fields necessary for fast retrieval of data. In this clause avoid adding too many indexes to keep INSERT performance high.
- Read Performance: Queries without indexes may be slower, but this is acceptable if the focus is on fast data entry.
- Write Performance: INSERT operations are faster without additional indexes since the database doesn’t need to update them for each new sale.
- Best for: Write-heavy applications, where data is constantly written, and fewer indexes are needed to keep insertion speeds high.
In read-heavy applications, more indexes can improve query speed, while in write-heavy applications, fewer indexes help maintain fast write performance. Balancing indexing based on your application’s read-write ratio is key to optimizing performance.
Materialized Views
A materialized view is a stored, precomputed result set based on a query that’s saved as a physical table. Unlike regular views, which are just stored queries that run in real time when accessed, materialized views persist the query result in storage. This means that when you query a materialized view, you’re querying data that has already been processed and stored, making it much faster to retrieve than recalculating the data each time.
Materialized views can significantly speed up complex, resource-intensive queries by reducing the need to repeatedly calculate results from large datasets. They are particularly useful for:
- Aggregations: Queries with complex aggregations (e.g., SUM, COUNT, AVG) benefit from materialized views because the aggregated data is precomputed.
Scenario: You have a sales table with columns sale_id, product_id, sale_date, and amount, and you frequently need to calculate the total sales amount per product. Every time you run this query, the database has to recalculate the SUM of amount for each product_id from the entire sales table, which can be slow for large datasets.
Create a materialized view that precomputes the total sales amount per product. This stores the results in a materialized view, so each query retrieves precomputed data instead of recalculating it.Step 1: Create the Materialized ViewSELECT product_id, SUM(amount) AS total_sales FROM sales GROUP BY product_id;
Step 2: Query the Materialized ViewCREATE MATERIALIZED VIEW product_sales_summary AS SELECT product_id, SUM(amount) AS total_sales FROM sales GROUP BY product_id;
Benefit: The query now retrieves precomputed totals from the materialized view, significantly reducing computation time.Refreshing the View: If data in the sales table changes, you can update the materialized view withSELECT * FROM product_sales_summary;
In PostgreSQL, materialized views do not automatically update when the underlying data changes. However, you can set up automatic refreshes by using triggers, cron jobs, or scheduled tasks.You can simulate near real-time updates by using a trigger function to refresh the view upon inserts, updates, or deletes in the underlying table.REFRESH MATERIALIZED VIEW product_sales_summary;
How it Works: This setup calls the refresh_sales_summary() function to refresh the view every time data in sales changes. Be cautious with this approach in write-heavy systems, as frequent refreshes can slow down performance.CREATE OR REPLACE FUNCTION refresh_sales_summary() RETURNS TRIGGER AS $$ BEGIN PERFORM pg_sleep(1); -- Optional delay to prevent excessive refreshing REFRESH MATERIALIZED VIEW product_sales_summary; RETURN NULL; END; $$ LANGUAGE plpgsql; CREATE TRIGGER sales_refresh_trigger AFTER INSERT OR UPDATE OR DELETE ON sales FOR EACH STATEMENT EXECUTE FUNCTION refresh_sales_summary();
- Joins on Large Tables: If you frequently join large tables, a materialized view with precomputed joins can save processing time.
Scenario:Suppose you have two large tables: orders and customers. Each time you want to view customer order details, you need to join these tables. Each time you query, the database performs a join on the entire orders and customers tables, which is resource-intensive.
Problem: For large tables, this query recalculates the join every time it runs, which is slow and resource-consuming.SELECT c.customer_name, o.order_date, o.amount FROM orders o JOIN customers c ON o.customer_id = c.customer_id WHERE o.order_date >= '2024-01-01';
Create a materialized view that precomputes the join between orders and customers. This way, the join operation only needs to be done once and can be quickly queried from the materialized view.Step 1: Create the Materialized View
Step 2: Query the Materialized ViewCREATE MATERIALIZED VIEW customer_order_summary AS SELECT c.customer_id, c.customer_name, o.order_date, o.amount FROM orders o JOIN customers c ON o.customer_id = c.customer_id;
Benefit: The materialized view precomputes and stores the join results, so queries can retrieve the data much faster without recalculating the join on large tables each time.SELECT customer_name, order_date, amount FROM customer_order_summary WHERE order_date >= '2024-01-01'; === REFRESH MATERIALIZED VIEW customer_order_summary;
- Frequent Reporting Queries: For reporting and analytics, where data does not need to be real-time, materialized views provide quick access to the data.
Scenario: Suppose you have a sales table with columns sale_id, product_id, sale_date, and amount. You frequently generate reports showing total sales per product for analysis. Each time you run this report, the database has to scan the entire sales table and calculate the SUM of amount for each product_id.
Problem: Running this aggregation directly on the sales table every time is slow and resource-intensive, especially with a large dataset. This approach is inefficient for frequent reports.SELECT product_id, SUM(amount) AS total_sales FROM sales GROUP BY product_id;
Create a materialized view that precomputes the total sales per product. This view will store the aggregated results, allowing you to query the data quickly without recalculating the totals each time.Step 1: Create the Materialized View
Step 2: Query the Materialized ViewCREATE MATERIALIZED VIEW product_sales_summary AS SELECT product_id, SUM(amount) AS total_sales FROM sales GROUP BY product_id;
Benefit: The materialized view precomputes the aggregation, so reporting queries can quickly access the pre-summarized data. This speeds up the report generation significantly.SELECT * FROM product_sales_summary; === REFRESH MATERIALIZED VIEW product_sales_summary;
- Staleness: Materialized views can become outdated if the underlying data changes frequently. Some databases support automatic refresh policies (e.g., refreshing the materialized view at intervals or based on specific triggers. Real-time refresh of a materialized view keeps it continuously updated with the latest data changes, ensuring near-instantaneous synchronization with the underlying tables.).
- Storage: Materialized views consume additional storage since they store the result set separately.
- Maintenance Overhead: Depending on the refresh method, maintaining up-to-date materialized views can add an overhead on write operations.
- No-relational databases (e.g., MongoDB) do not support materialized views. However, you can implement a materialized view-like solution by using aggregation pipelines, storing aggregated results in a new collection, and updating it periodically.
In essence, optimizing database queries and indexing involves carefully structuring queries to retrieve only the necessary data in an efficient way, and using indexes strategically to improve retrieval speed without overloading the database. These practices are essential for maintaining high performance, especially in applications with large datasets or high user traffic.
Denormalization
Denormalization is a distinct database optimization technique focused on improving read performance by reducing the need for JOINS or NESTED QUERIES. While it can be used alongside query optimization and indexing, it requires careful handling to balance performance gains with the potential for data inconsistency and increased storage requirements. For applications with high read demands and relatively stable data, denormalization can be an effective strategy, making it a valuable part of the toolkit for database performance tuning.
Normalization vs. Denormalization
- In a normalized database (OLTP - Online Transaction Processing), data is organized into multiple tables to reduce redundancy and ensure data integrity. Each table usually has a single responsibility, and relationships between tables are managed through foreign keys. For instance, in a normalized e-commerce database, customer details might be in one table, orders in another, and products in a third. To retrieve customer orders, a query would need to join these tables.
Scenario: In a normalized e-commerce database, customers, orders, and products are stored in separate tables. To retrieve customer orders, a query needs to join these tables. To get all orders placed by a specific customer (e.g., customer with customer_id = 123), including product details:SELECT c.name AS customer_name, o.order_id, o.order_date, p.product_name, oi.quantity, oi.price FROM customers c JOIN orders o ON c.customer_id = o.customer_id JOIN order_items oi ON o.order_id = oi.order_id JOIN products p ON oi.product_id = p.product_id WHERE c.customer_id = 123;
- In denormalization (OLAP- Online Analytical Processing), data that is frequently accessed together is stored together in a single table, even if it creates redundancy. For example, customer details might be duplicated in the orders table to avoid joining tables when querying customer order history.
Scenario: In a denormalized e-commerce database, data frequently accessed together (e.g., customer and order details) is stored in a single table, even if it creates redundancy. To get all orders placed by a specific customer (e.g., customer with customer_name = 'John Doe'):SELECT customer_name, order_id, order_date, product_name, quantity, price, total_amount FROM orders_summary WHERE customer_name = 'John Doe';
When to Use Denormalization
- Read-heavy applications: If the application performs many read operations but few write operations, denormalization can help improve query performance.
- High latency from joins: If multiple joins slow down queries significantly, denormalization reduces or eliminates the need for them.
- Precomputed Aggregations: For large datasets where aggregations or calculations (such as sums or averages) are frequently needed, denormalizing by storing precomputed values speeds up access.
Trade-offs and Drawbacks
- Data Redundancy: Denormalization increases data redundancy, meaning the same data is stored in multiple places. This leads to higher storage usage.
- Data Inconsistency: Maintaining consistent data across multiple tables can be challenging. If a change is made to a duplicated field in one table, all other tables holding that data must be updated.
- Slower Write Performance: Write operations (inserts, updates, deletes) can be slower, as any change to data must be applied to multiple places.
Vertical Scaling (Scaling Up)
Vertical Scaling (Scaling Up) is a method of increasing the capacity of a single server or machine to handle a larger load. Instead of adding more servers, vertical scaling focuses on enhancing the existing hardware by upgrading its resources, such as CPU, RAM, and storage. This approach allows the same database instance to handle more transactions and support more concurrent users.
Horizontal Scaling / Replication (Scaling Out)
In a horizontally scaled web application, multiple servers host the same application. When user traffic increases, additional servers are added, and a load balancer directs user requests to different servers. Each server handles a subset of users, helping maintain fast response times even as the application scales.
In summary, Horizontal Scaling (Scaling Out) provides a flexible, resilient way to handle growing demand by expanding infrastructure outward, making it a preferred choice for high-availability applications and large-scale databases.
Conclusion
Scaling databases for high-traffic applications is both an art and a science, requiring a deep understanding of your application’s needs, database capabilities, and traffic patterns. The strategies outlined in this article — from optimizing queries, indexing, and leveraging materialized views to adopting denormalization techniques and choosing the right scaling approach (vertical vs. horizontal) — equip developers and database administrators with a robust toolkit to tackle performance challenges.
Effective database scaling ensures not only the stability and reliability of your application but also enhances the user experience by delivering faster and more efficient responses. It’s important to remember that no single strategy fits all scenarios. Balancing trade-offs such as storage costs, maintenance overhead, and system complexity is key to achieving the right mix of scalability and performance.
Stay tuned for Part 2, where we’ll explore advanced strategies like database partitioning, replication, sharding, and caching, along with practical examples to further enhance your ability to scale and optimize databases for demanding applications.