How to Build and Run a Neural Network with TensorFlow - Complete Tutorial
In this tutorial, I walk you through building and running a simple neural network using TensorFlow for binary classification. Whether you're new to machine learning or just starting with TensorFlow, this guide is perfect for you! We'll cover everything from setting up your environment, loading a dataset, and splitting it into training and testing sets, to building and training your neural network. Finally, we’ll evaluate the model and make predictions. By the end, you'll have a working model and understand how to create your own neural network projects!
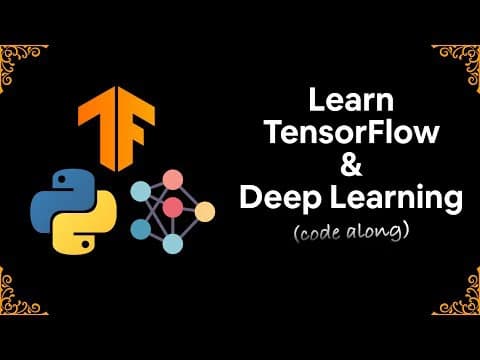
Imports
print("
Predictions vs Actuals:")
for i in range(len(predictions_binary)):
print(f"Predicted: {predictions_binary[i]}, Actual: {y_test.iloc[i]}")
- TensorFlow (tf): This is the primary library used to build and train neural networks.
- Pandas (pd): This is used for loading and manipulating datasets. In this case, it's used to manage a simple dataset.
- train_test_split: A function from Scikit-Learn used to split the dataset into training and testing subsets.
- StandardScaler: Another Scikit-Learn tool used to normalize/standardize feature values so they have a mean of 0 and a standard deviation of 1.
- os.environ['TF_CPP_MIN_LOG_LEVEL'] = '3': This setting suppresses unnecessary TensorFlow log messages for a cleaner output.
Dataset Creation
data = {
'age': [25, 32, 47, 51, 62, 23, 56, 45, 36, 28],
'salary': [50000, 60000, 120000, 90000, 150000, 45000, 130000, 100000, 85000, 62000],
'years_of_membership': [1, 4, 9, 7, 11, 2, 10, 8, 5, 3],
'interest_in_product': [1, 1, 1, 0, 0, 1, 1, 0, 0, 1],
'purchased': [0, 1, 1, 0, 1, 0, 1, 0, 0, 1] # Target variable (0 = No, 1 = Yes)
}
df = pd.DataFrame(data)
- A fictitious dataset is created manually with columns such as age, salary, years_of_membership, interest_in_product, and the target variable purchased (whether or not a product was purchased).
- This dataset is converted into a Pandas DataFrame for easy manipulation.
Splitting Features and Target Variable
x = df.drop('purchased', axis=1) # Features (independent variables)
y = df['purchased'] # Target (dependent variable)
- x contains the features (age, salary, etc.) used to predict whether a customer will make a purchase.
- y is the target variable, representing whether a purchase was made (purchased column).
Splitting the Dataset into Training and Test Sets
x_train, x_test, y_train, y_test = train_test_split(x, y, test_size=0.2, random_state=42)
The dataset is split into training and test sets.
- 80% of the data is used for training.
- 20% of the data is used for testing.
- random_state=42 ensures that the split is reproducible.
Feature Normalization
scaler = StandardScaler()
x_train_scaled = scaler.fit_transform(x_train)
x_test_scaled = scaler.transform(x_test)
- StandardScaler is used to normalize the feature values so that they have a mean of 0 and a standard deviation of 1.
- This normalization ensures that no one feature (like salary) disproportionately affects the model.
- fit_transform is applied on the training data, and transform is applied on the test data (without fitting again).
Building the Neural Network Model
model = tf.keras.Sequential([
tf.keras.layers.Dense(8, input_shape=(4,), activation='relu'), # Hidden layer with 8 neurons
tf.keras.layers.Dense(1, activation='sigmoid') # Output layer with 1 neuron (for binary classification)
])
A simple feed-forward neural network (sequential model) is built:
- First layer: A hidden layer with 8 neurons and the ReLU (rectified linear unit) activation function.
- Second layer: The output layer with 1 neuron (since it's binary classification), using a sigmoid activation function to output a probability between 0 and 1.
Compiling the Model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
The model is compiled with:
- Optimizer: adam, which is an adaptive learning rate method that's widely used in deep learning.
- Loss function: binary_crossentropy, which is appropriate for binary classification tasks.
- Metric: accuracy, which will be used to track performance during training and evaluation.
Training the Model
history = model.fit(x_train_scaled, y_train, epochs=50, batch_size=2, verbose=1)
The model is trained on the training data:
- 50 epochs: The number of times the model will go through the entire training dataset.
- Batch size of 2: The number of samples used to update the model's weights before moving on to the next batch.
- verbose=1 means that progress updates will be shown during training.
Evaluating the Model
test_loss, test_acc = model.evaluate(x_test_scaled, y_test, verbose=1)
print(f'
Test Accuracy: {test_acc:.2f}')
- The model is evaluated on the test data to see how well it generalizes to unseen data.
- evaluate outputs the test loss and test accuracy, and the accuracy is printed.
Making Predictions
predictions = model.predict(x_test_scaled)
# Convert probabilities to binary outputs (0 or 1)
predictions_binary = [1 if prob > 0.5 else 0 for prob in predictions]
- The model is used to predict probabilities for each test sample (between 0 and 1).
- These probabilities are then converted into binary predictions (0 or 1) using a threshold of 0.5.
Comparing Predictions with Actual Values
predictions = model.predict(x_test_scaled)
# Convert probabilities to binary outputs (0 or 1)
predictions_binary = [1 if prob > 0.5 else 0 for prob in predictions]
- The predicted values (binary) are compared with the actual values from the test set, and each comparison is printed out for inspection.
This script creates, trains, and evaluates a simple binary classification neural network using a small, synthetic dataset.
https://github.com/cholakovit/customer_purchase_prediction